输入一个字符串,删除其中出现两次的字符
时间: 2023-05-28 20:01:53 浏览: 63
这道题有点模糊,是删除所有出现两次及以上的字符还是仅删除两次的字符呢?下面提供两种方法,可根据实际情况选择:
方法一:删除所有出现两次及以上的字符
思路:遍历字符串,使用字典记录每个字符出现的次数,最后删除出现次数大于等于2的字符。
实现代码:
```python
def remove_duplicate_chars(s):
count = {}
for c in s:
if c in count:
count[c] += 1
else:
count[c] = 1
new_s = ""
for c in s:
if count[c] < 2:
new_s += c
return new_s
```
测试:
```python
s = "aabbcdeeffgg"
print(remove_duplicate_chars(s)) # "cdef"
```
方法二:删除仅出现两次的字符
思路:遍历字符串,记录每个字符出现的位置,最后删除出现次数为2且位置相同的字符。
实现代码:
```python
def remove_duplicate_chars(s):
count = {}
positions = {}
for i, c in enumerate(s):
if c in count:
count[c] += 1
positions[c].append(i)
else:
count[c] = 1
positions[c] = [i]
new_s = ""
for c, cnt in count.items():
if cnt != 2: # 出现次数不为2的字符直接加入新字符串
new_s += c
else: # 出现次数为2的字符需要判断位置
if positions[c][0] != positions[c][1]:
new_s += c
return new_s
```
测试:
```python
s = "aabbcdeeffgg"
print(remove_duplicate_chars(s)) # "abcdeffgg"
```
相关推荐
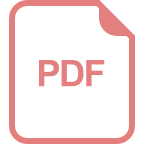
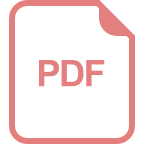
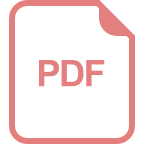

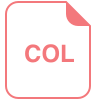
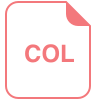
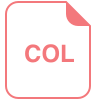
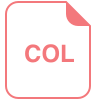









