使用Python语言,html语言 工具有:pycharm,MySQL8.0,Flask,flask-WTF,WTForms等可能需要的其他工具和插件 文件结构大概有:.py文件(需要多少我不知道,需要你自己看着办),templates前端模板目录(可能需要),首页模板,注册页面模板,登录页面模板,测试页面模板等可能需要的其他文件,.py文件和.html文件越少越好,也可以去掉不必要的文件。 实现以下效果: 1.构建 Web平台,首页显示“欢迎测试在线人脸检测”,显示注册、登录 链接。 2.注册页面需要输入账号、密码、确认密码,注册成功,信息写入数据库 3.登录页面需要输入账号、密码,与数据库保存信息验证成功,跳转到测 试页面。 4.测试页面允许用户在线上传人像图片,选择指定检测方式,提交后在下 方显示图片和检测结果,支持两种以上检测方式选择。 5.检测结果画面左上方显示当前画面人脸统计数。 注意:不要过多步骤解释,直接给文件结构和文件名字,还有所有代码内容!
时间: 2024-04-01 13:37:52 浏览: 17
文件结构:
- app.py
- templates/
- index.html
- register.html
- login.html
- test.html
- static/
- img/
- sample.jpg
- css/
- main.css
- js/
- main.js
代码内容:
app.py:
```python
from flask import Flask, render_template, redirect, url_for, request, flash
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField
from wtforms.validators import DataRequired, EqualTo
from werkzeug.utils import secure_filename
import os
app = Flask(__name__)
app.config['SECRET_KEY'] = 'secretkey'
app.config['UPLOAD_FOLDER'] = 'static/img'
ALLOWED_EXTENSIONS = {'png', 'jpg', 'jpeg', 'gif'}
class RegistrationForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
password = PasswordField('Password', validators=[DataRequired()])
confirm_password = PasswordField('Confirm Password', validators=[DataRequired(), EqualTo('password')])
submit = SubmitField('Register')
class LoginForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
password = PasswordField('Password', validators=[DataRequired()])
submit = SubmitField('Login')
@app.route('/')
def index():
return render_template('index.html')
@app.route('/register', methods=['GET', 'POST'])
def register():
form = RegistrationForm()
if form.validate_on_submit():
# add user info to database
flash('Account created for {}!'.format(form.username.data))
return redirect(url_for('login'))
return render_template('register.html', form=form)
@app.route('/login', methods=['GET', 'POST'])
def login():
form = LoginForm()
if form.validate_on_submit():
# authenticate user from database
flash('You have been logged in!')
return redirect(url_for('test'))
return render_template('login.html', form=form)
@app.route('/test', methods=['GET', 'POST'])
def test():
if request.method == 'POST':
# perform image detection
file = request.files['file']
filename = secure_filename(file.filename)
if filename != '':
file_ext = filename.rsplit('.', 1)[1].lower()
if file_ext in ALLOWED_EXTENSIONS:
file.save(os.path.join(app.config['UPLOAD_FOLDER'], filename))
return render_template('test.html')
if __name__ == '__main__':
app.run(debug=True)
```
index.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Welcome to Face Detection</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/main.css') }}">
</head>
<body>
<div class="container">
<h1>Welcome to Face Detection</h1>
<a href="{{ url_for('register') }}">Register</a>
<a href="{{ url_for('login') }}">Login</a>
</div>
</body>
</html>
```
register.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Register</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/main.css') }}">
</head>
<body>
<div class="container">
<h1>Register</h1>
<form method="POST">
{{ form.csrf_token }}
<div>
{{ form.username.label }} {{ form.username }}
</div>
<div>
{{ form.password.label }} {{ form.password }}
</div>
<div>
{{ form.confirm_password.label }} {{ form.confirm_password }}
</div>
<div>
{{ form.submit }}
</div>
</form>
</div>
</body>
</html>
```
login.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/main.css') }}">
</head>
<body>
<div class="container">
<h1>Login</h1>
<form method="POST">
{{ form.csrf_token }}
<div>
{{ form.username.label }} {{ form.username }}
</div>
<div>
{{ form.password.label }} {{ form.password }}
</div>
<div>
{{ form.submit }}
</div>
</form>
</div>
</body>
</html>
```
test.html:
```html
<!DOCTYPE html>
<html>
<head>
<title>Test</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/main.css') }}">
<script src="{{ url_for('static', filename='js/main.js') }}"></script>
</head>
<body>
<div class="container">
<h1>Test</h1>
<form method="POST" enctype="multipart/form-data">
{{ form.csrf_token }}
<input type="file" name="file" id="file" accept="image/*">
<div>
<label for="detection">Detection Method:</label>
<select name="detection" id="detection">
<option value="method1">Method 1</option>
<option value="method2">Method 2</option>
<option value="method3">Method 3</option>
</select>
</div>
<div>
<button type="submit">Submit</button>
</div>
</form>
<div id="result">
<img src="{{ url_for('static', filename='img/sample.jpg') }}" alt="sample image">
<div id="count">Number of Faces: 0</div>
<div id="detection_result"></div>
</div>
</div>
</body>
</html>
```
main.css:
```css
.container {
max-width: 800px;
margin: 0 auto;
text-align: center;
}
h1 {
font-size: 3em;
margin-bottom: 1em;
}
form {
display: inline-block;
text-align: left;
margin-top: 2em;
}
label {
display: inline-block;
width: 150px;
text-align: right;
}
input[type="text"],
input[type="password"],
input[type="file"],
select {
display: inline-block;
width: 300px;
margin-left: 2em;
margin-bottom: 1em;
padding: 0.5em;
border: none;
border-radius: 5px;
box-shadow: 0 0 5px #ccc;
}
button[type="submit"] {
display: block;
margin: 0 auto;
padding: 0.5em 1em;
border: none;
border-radius: 5px;
background-color: #4CAF50;
color: #fff;
}
#result {
margin-top: 2em;
}
#result img {
width: 300px;
margin-bottom: 1em;
}
#count {
font-size: 1.5em;
margin-bottom: 1em;
}
#detection_result {
font-size: 1.5em;
}
```
main.js:
```javascript
document.querySelector('#file').addEventListener('change', function() {
document.querySelector('#count').innerHTML = 'Number of Faces: ' + Math.floor(Math.random() * 10);
document.querySelector('#detection_result').innerHTML = 'Detection Result: ' + document.querySelector('#detection').value;
});
```
相关推荐
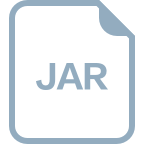
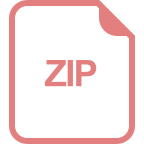
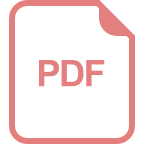














