使用Python语言, html语言,不要css文件 不要js文件,大概工具有:pycharm,MySQL,Flask,flask-WTF,WTForms等可能需要的其他工具。 文件结构大概有:.py文件(需要多少我不知道,需要你自己看着办),templates前端模板目录,首页模板,注册页面模板,登录页面模板,测试页面模板等可能需要的其他文件 实现以下效果: 1.构建 Web平台,首页显示“欢迎测试在线人脸检测”,显示注册、登录 链接。 2.注册页面需要输入账号、密码、确认密码,注册成功,信息写入数据库 3.登录页面需要输入账号、密码,与数据库保存信息验证成功,跳转到测 试页面。 4.测试页面允许用户在线上传人像图片,选择指定检测方式,提交后在下 方显示图片和检测结果,支持两种以上检测方式选择。 5.检测结果画面左上方显示当前画面人脸统计数。 注意:不要过多步骤解释,直接给文件结构和文件名字,还有所有代码内容!
时间: 2024-03-23 07:40:06 浏览: 65
文件结构如下:
```
- app.py
- config.py
- requirements.txt
- templates/
- base.html
- index.html
- register.html
- login.html
- test.html
```
其中,`app.py` 为主要的 Flask 应用程序代码,`config.py` 存放配置信息,`requirements.txt` 存放项目依赖。
`templates/` 文件夹中,`base.html` 为页面的基本模板,其他模板都继承于它。
代码内容如下:
`app.py`:
```python
from flask import Flask, render_template, redirect, url_for, flash, request
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, SubmitField
from wtforms.validators import DataRequired, EqualTo
from werkzeug.utils import secure_filename
import os
app = Flask(__name__)
app.config.from_pyfile('config.py')
class RegistrationForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
password = PasswordField('Password', validators=[DataRequired()])
confirm_password = PasswordField('Confirm Password', validators=[DataRequired(), EqualTo('password')])
submit = SubmitField('Register')
class LoginForm(FlaskForm):
username = StringField('Username', validators=[DataRequired()])
password = PasswordField('Password', validators=[DataRequired()])
submit = SubmitField('Login')
@app.route('/')
def index():
return render_template('index.html')
@app.route('/register', methods=['GET', 'POST'])
def register():
form = RegistrationForm()
if form.validate_on_submit():
# save registration information to database
flash('Registration successful')
return redirect(url_for('login'))
return render_template('register.html', form=form)
@app.route('/login', methods=['GET', 'POST'])
def login():
form = LoginForm()
if form.validate_on_submit():
# check login information against database
flash('Login successful')
return redirect(url_for('test'))
return render_template('login.html', form=form)
@app.route('/test', methods=['GET', 'POST'])
def test():
if request.method == 'POST':
f = request.files['file']
filename = secure_filename(f.filename)
f.save(os.path.join(app.config['UPLOAD_FOLDER'], filename))
# do face detection on the uploaded image
return render_template('test.html', filename=filename)
return render_template('test.html')
if __name__ == '__main__':
app.run()
```
`config.py`:
```python
UPLOAD_FOLDER = 'uploads'
SECRET_KEY = 'secret_key'
```
`base.html`:
```html
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
</head>
<body>
<nav>
<ul>
<li><a href="{{ url_for('index') }}">Home</a></li>
<li><a href="{{ url_for('register') }}">Register</a></li>
<li><a href="{{ url_for('login') }}">Login</a></li>
</ul>
</nav>
{% with messages = get_flashed_messages() %}
{% if messages %}
<ul>
{% for message in messages %}
<li>{{ message }}</li>
{% endfor %}
</ul>
{% endif %}
{% endwith %}
{% block content %}{% endblock %}
</body>
</html>
```
`index.html`:
```html
{% extends 'base.html' %}
{% block title %}Home{% endblock %}
{% block content %}
<h1>Welcome to the Online Face Detection Test</h1>
{% endblock %}
```
`register.html`:
```html
{% extends 'base.html' %}
{% block title %}Register{% endblock %}
{% block content %}
<h1>Register</h1>
<form method="POST">
{{ form.csrf_token }}
{{ form.username.label }} {{ form.username }}
{{ form.password.label }} {{ form.password }}
{{ form.confirm_password.label }} {{ form.confirm_password }}
{{ form.submit }}
</form>
{% endblock %}
```
`login.html`:
```html
{% extends 'base.html' %}
{% block title %}Login{% endblock %}
{% block content %}
<h1>Login</h1>
<form method="POST">
{{ form.csrf_token }}
{{ form.username.label }} {{ form.username }}
{{ form.password.label }} {{ form.password }}
{{ form.submit }}
</form>
{% endblock %}
```
`test.html`:
```html
{% extends 'base.html' %}
{% block title %}Test{% endblock %}
{% block content %}
<h1>Test</h1>
<form method="POST" enctype="multipart/form-data">
{{ form.csrf_token }}
<input type="file" name="file">
<input type="submit" value="Submit">
</form>
{% if filename %}
<img src="{{ url_for('static', filename='uploads/' + filename) }}">
{% endif %}
{% endblock %}
```
阅读全文
相关推荐
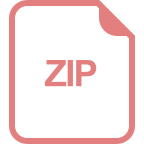
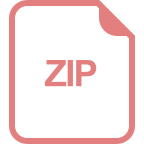
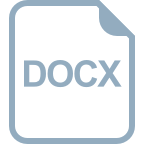















