两个简单矩阵相加,如何实现一个类来进行运算符重载
时间: 2024-01-27 11:03:42 浏览: 66
实现两个简单矩阵相加的关键是要定义一个矩阵类,并在该类中重载加法运算符"+"。下面是一个简单的实现示例:
```
#include<iostream>
using namespace std;
class Matrix {
private:
int m_rows;
int m_cols;
int** m_data;
public:
Matrix(int rows, int cols) :m_rows(rows), m_cols(cols) {
// 动态分配二维数组
m_data = new int*[m_rows];
for (int i = 0; i < m_rows; i++) {
m_data[i] = new int[m_cols];
}
}
// 拷贝构造函数
Matrix(const Matrix& other) {
m_rows = other.m_rows;
m_cols = other.m_cols;
// 动态分配二维数组
m_data = new int*[m_rows];
for (int i = 0; i < m_rows; i++) {
m_data[i] = new int[m_cols];
for (int j = 0; j < m_cols; j++) {
m_data[i][j] = other.m_data[i][j];
}
}
}
// 析构函数
~Matrix() {
// 释放动态分配的内存
for (int i = 0; i < m_rows; i++) {
delete[] m_data[i];
}
delete[] m_data;
}
// 重载加法运算符"+"
Matrix operator+(const Matrix& other) const {
if (m_rows != other.m_rows || m_cols != other.m_cols) {
// 两个矩阵大小不一致,无法相加
throw "Matrix size not match";
}
Matrix result(m_rows, m_cols);
for (int i = 0; i < m_rows; i++) {
for (int j = 0; j < m_cols; j++) {
result.m_data[i][j] = m_data[i][j] + other.m_data[i][j];
}
}
return result;
}
// 重载输出运算符"<<"
friend ostream& operator<<(ostream& os, const Matrix& matrix) {
for (int i = 0; i < matrix.m_rows; i++) {
for (int j = 0; j < matrix.m_cols; j++) {
os << matrix.m_data[i][j] << " ";
}
os << endl;
}
return os;
}
// 重载输入运算符">>"
friend istream& operator>>(istream& is, Matrix& matrix) {
for (int i = 0; i < matrix.m_rows; i++) {
for (int j = 0; j < matrix.m_cols; j++) {
is >> matrix.m_data[i][j];
}
}
return is;
}
};
int main() {
// 定义两个3*3的矩阵对象
Matrix m1(3, 3), m2(3, 3);
// 从标准输入流中读入这两个矩阵对象的元素值
cin >> m1 >> m2;
// 将这两个矩阵对象相加得到结果矩阵
Matrix result = m1 + m2;
// 将结果矩阵输出到标准输出流中
cout << result;
return 0;
}
```
在上面的代码中,我们定义了一个名为Matrix的矩阵类,其中包含了矩阵的行数、列数和元素数组,以及一些成员函数,如构造函数、拷贝构造函数、析构函数、重载加法运算符"+"、重载输出运算符"<<"和重载输入运算符">>"。
在主函数中,我们首先定义了两个3*3的Matrix对象m1和m2,然后通过输入运算符">>"从标准输入流中读入这两个矩阵对象的元素值,然后通过重载的加法运算符"+"将这两个矩阵对象相加得到结果矩阵,最后通过输出运算符"<<"将结果矩阵输出到标准输出流中。
需要注意的是,在重载加法运算符"+"时,我们返回了一个新的Matrix对象result,该对象的元素值为两个矩阵对象对应位置的和。另外,在重载输入运算符">>"和输出运算符"<<"时,我们使用了友元函数的方式,这样可以访问到类的私有成员变量。
阅读全文
相关推荐
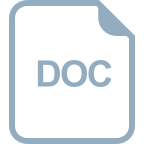
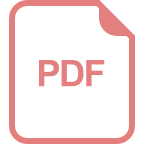





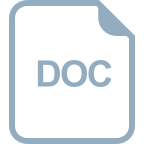
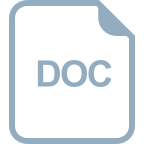







