编写一个c++ 定义一个矩阵类array重载求两个矩阵的和
时间: 2023-11-25 21:08:26 浏览: 132
好的,下面是一个简单的矩阵类的定义,包含矩阵加法的重载:
```c++
#include <iostream>
#include <vector>
using namespace std;
class Array {
public:
Array(int rows, int cols) : m_rows(rows), m_cols(cols), m_data(rows * cols) {}
int rows() const { return m_rows; }
int cols() const { return m_cols; }
int& operator()(int row, int col) {
return m_data[row * m_cols + col];
}
const int& operator()(int row, int col) const {
return m_data[row * m_cols + col];
}
Array operator+(const Array& other) const {
if (m_rows != other.m_rows || m_cols != other.m_cols) {
throw invalid_argument("Arrays must have the same dimensions");
}
Array result(m_rows, m_cols);
for (int i = 0; i < m_rows; ++i) {
for (int j = 0; j < m_cols; ++j) {
result(i, j) = (*this)(i, j) + other(i, j);
}
}
return result;
}
private:
int m_rows;
int m_cols;
vector<int> m_data;
};
int main() {
Array a(2, 2);
a(0, 0) = 1;
a(0, 1) = 2;
a(1, 0) = 3;
a(1, 1) = 4;
Array b(2, 2);
b(0, 0) = 5;
b(0, 1) = 6;
b(1, 0) = 7;
b(1, 1) = 8;
Array c = a + b;
for (int i = 0; i < c.rows(); ++i) {
for (int j = 0; j < c.cols(); ++j) {
cout << c(i, j) << " ";
}
cout << endl;
}
return 0;
}
```
这个类包含了一个构造函数,一个 rows() 和 cols() 方法用于获取矩阵的维度,一个重载了 () 运算符的方法用于访问矩阵中的元素,以及一个重载了 + 运算符的方法用于计算两个矩阵的和。在 main 函数中,我们创建了两个 2x2 的矩阵 a 和 b,将它们相加,并打印出结果矩阵 c。
阅读全文
相关推荐
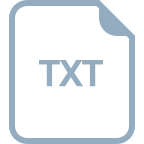
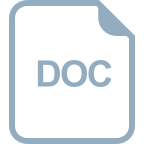
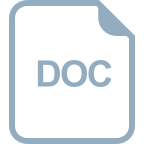








