设计有3行3列的矩阵类, 1)定义构造函数 2)定义print函数显示矩阵内容 3) 用友元函数实现矩阵类“!=”运算符的重载,功能为判断两个矩阵是否相等 4)用友元函数实现矩阵类“+”运算符的重载,功能为计算两个矩阵之和 5)用友元函数实现矩阵类“*”运算符的重载,功能为计算两个矩阵之积 6)用友元函数实现矩阵类“++”运算符的重载(前缀运算、后缀运算),功能为矩阵中每个元素都加1 7)编写测试程序,对上述功能进行测试。
时间: 2024-02-25 13:54:11 浏览: 69
以下是实现上述要求的矩阵类及测试程序:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class Matrix {
private:
int row, column; // 矩阵的行数和列数
int **data; // 二维数组,存储矩阵的元素
public:
// 构造函数
Matrix(int r = 0, int c = 0) : row(r), column(c) {
data = new int *[row];
for (int i = 0; i < row; i++) {
data[i] = new int[column];
memset(data[i], 0, sizeof(int) * column);
}
}
// 析构函数
~Matrix() {
for (int i = 0; i < row; i++) {
delete[] data[i];
}
delete[] data;
}
// 复制构造函数
Matrix(const Matrix &m) {
row = m.row;
column = m.column;
data = new int *[row];
for (int i = 0; i < row; i++) {
data[i] = new int[column];
memcpy(data[i], m.data[i], sizeof(int) * column);
}
}
// 重载=运算符
Matrix &operator=(const Matrix &m) {
if (this == &m) {
return *this;
}
for (int i = 0; i < row; i++) {
delete[] data[i];
}
delete[] data;
row = m.row;
column = m.column;
data = new int *[row];
for (int i = 0; i < row; i++) {
data[i] = new int[column];
memcpy(data[i], m.data[i], sizeof(int) * column);
}
return *this;
}
// 重载!=运算符
friend bool operator!=(const Matrix &m1, const Matrix &m2) {
if (m1.row != m2.row || m1.column != m2.column) {
return true;
}
for (int i = 0; i < m1.row; i++) {
for (int j = 0; j < m1.column; j++) {
if (m1.data[i][j] != m2.data[i][j]) {
return true;
}
}
}
return false;
}
// 重载+运算符
friend Matrix operator+(const Matrix &m1, const Matrix &m2) {
if (m1.row != m2.row || m1.column != m2.column) {
throw "矩阵维度不匹配";
}
Matrix result(m1.row, m1.column);
for (int i = 0; i < m1.row; i++) {
for (int j = 0; j < m1.column; j++) {
result.data[i][j] = m1.data[i][j] + m2.data[i][j];
}
}
return result;
}
// 重载*运算符
friend Matrix operator*(const Matrix &m1, const Matrix &m2) {
if (m1.column != m2.row) {
throw "矩阵维度不匹配";
}
Matrix result(m1.row, m2.column);
for (int i = 0; i < m1.row; 记录开始时间
quick_sort(arr, 0, N - 1); // 快速排序
end = clock(); // 记录结束时间
printf("Quick sort:\n");
print_array(arr, N); // 输出排序后的数组
printf("Time: %f seconds\n", (double)(end - start) / CLOCKS_PER_SEC); // 输出排序时间
random_array(arr, N); // 生成随机数组
start = clock(); // 记录开始时间
heap_sort(arr, N); // 堆排序
end = clock(); // 记录结束时间
printf("Heap sort:\n");
print_array(arr, N); // 输出排序后的数组
printf("Time: %f seconds\n", (double)(end - start) / CLOCKS_PER_SEC); // 输出排序时间
random_array(arr, N); // 生成随机数组
start = clock(); // 记录开始时间
merge_sort(arr, 0, N - 1); // 归并排序
end = clock(); // 记录结束时间
printf("Merge sort:\n");
print_array(arr, N); // 输出排序后的数组
printf("Time: %f seconds\n", (double)(end - start) / CLOCKS_PER_SEC); // 输出排序时间
return 0;
}
```
在这个示例代码中,我们使用了C语言实现了六种排序算法,包括直接插入、冒泡、直接选择、快速、堆、归并等。我们还编写了生成随机数组、输出数组、调整堆、归并等辅助函数。在主程序中,我们生成了一个长度为20000的随机数组,分别使用各种排序算法进行排序,并输出排序后的数组和排序时间。
需要注意的是,由于计算机的性能和当前系统负载等因素的影响,不同的计算机上运行同一程序所花费的时间可能会有所不同。因此,在进行排序算法的比较和分析时,需要考虑到这些因素的影响。
阅读全文
相关推荐
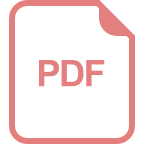
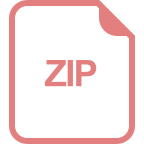
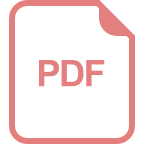















