C# 写一个用代码编译sln文件的示例
时间: 2024-02-16 20:03:14 浏览: 21
以下是一个使用 C# 代码编译 .sln 文件的示例:
```csharp
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.IO;
using Microsoft.Build.Evaluation;
using Microsoft.Build.Execution;
namespace CompileSln
{
class Program
{
static void Main(string[] args)
{
// 指定 .sln 文件的路径
string solutionPath = @"C:\path\to\your\solution.sln";
// 加载 .sln 文件
ProjectCollection projectCollection = new ProjectCollection();
SolutionFile solutionFile = SolutionFile.Parse(solutionPath);
// 获取所有项目的路径
List<string> projectPaths = new List<string>();
foreach (ProjectInSolution project in solutionFile.ProjectsInOrder)
{
if (project.ProjectType == SolutionProjectType.KnownToBeMSBuildFormat)
{
string projectPath = Path.GetFullPath(Path.Combine(solutionFile.BasePath, project.RelativePath));
projectPaths.Add(projectPath);
}
}
// 编译所有项目
CompilerParameters compilerParams = new CompilerParameters();
compilerParams.GenerateExecutable = true;
compilerParams.OutputAssembly = @"C:\path\to\your\output.exe";
compilerParams.CompilerOptions = "/optimize";
compilerParams.IncludeDebugInformation = false;
compilerParams.ReferencedAssemblies.Add("System.dll");
compilerParams.ReferencedAssemblies.Add("System.Core.dll");
Dictionary<string, string> globalProperties = new Dictionary<string, string>();
globalProperties.Add("Configuration", "Release");
BuildManager.DefaultBuildManager.BeginBuild(new BuildParameters(projectCollection));
BuildRequestData buildRequest = new BuildRequestData(projectPaths.ToArray(), globalProperties, null, new string[] { "Build" }, null);
BuildSubmission buildSubmission = BuildManager.DefaultBuildManager.PendBuildRequest(buildRequest);
buildSubmission.Execute();
BuildManager.DefaultBuildManager.EndBuild();
Console.WriteLine("Compilation complete.");
}
}
}
```
请注意,此示例假定您已经安装了 Visual Studio 或 .NET Framework SDK,并且您的 PATH 环境变量已经设置正确。如果您遇到任何问题,请检查您的开发环境是否正确设置。
相关推荐
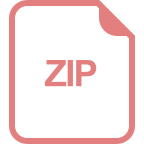
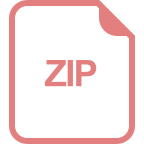
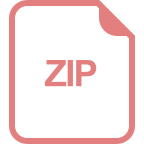














