c++类为什么可以将将函数声明放到private中
时间: 2023-06-13 07:09:06 浏览: 152
C++中的类可以将函数声明放到private中,这是因为C++中的访问控制机制允许类的成员函数只能被类的内部访问,而不能被类的外部访问。将函数声明放到private中可以实现这种访问控制的效果,即只有类的成员函数才能访问该函数,而类的外部代码无法访问该函数。
另外,将函数声明放到private中可以实现信息隐藏的效果,即将类的内部实现细节隐藏起来,只向外界暴露必要的接口。这样可以提高代码的可维护性和安全性。
相关问题
创建一个employee类,该类中有姓名、城市、邮政编码等数据成员。成员函数setValue设定对象数据,display函数输出对象数据。 具体要求如下: (1)数据成员设定成private (2)成员函数设定成public (3)建立指针对象,利用指针对象对成员进行引用 (4)将类声明和成员函数的定义分开,放到.h和.cpp中
好的,我来回答你的问题。
首先,这是一个关于C++面向对象编程的问题。我们需要创建一个employee类,具体实现如下:
在employee.h文件中定义如下:
```cpp
#ifndef EMPLOYEE_H
#define EMPLOYEE_H
#include <string>
class Employee {
public:
// 构造函数
Employee() {}
// 成员函数
void setValue(const std::string& name, const std::string& city, const std::string& zipCode);
void display();
private:
// 数据成员
std::string m_name;
std::string m_city;
std::string m_zipCode;
};
#endif // EMPLOYEE_H
```
在employee.cpp文件中定义如下:
```cpp
#include "employee.h"
#include <iostream>
using namespace std;
void Employee::setValue(const std::string& name, const std::string& city, const std::string& zipCode) {
m_name = name;
m_city = city;
m_zipCode = zipCode;
}
void Employee::display() {
cout << "Name: " << m_name << endl;
cout << "City: " << m_city << endl;
cout << "Zip Code: " << m_zipCode << endl;
}
```
然后,在main函数中,我们可以创建一个指针对象,对成员进行引用,具体实现如下:
```cpp
#include "employee.h"
int main() {
Employee* emp = new Employee();
emp->setValue("Tom", "Beijing", "100000");
emp->display();
delete emp;
return 0;
}
```
这样,我们就完成了一个简单的employee类的定义和使用。注意,我们将类的声明和成员函数的定义分开,放到了.h和.cpp文件中,这是面向对象编程的一种良好的习惯。
2.创建student类,在main函数中输入3个学生数据,包括学号,姓名和成绩,要求只输出成绩在80~90分数段的学生数据。 具体要求如下: (1)数据成员设定成private (2)成员函数设定成public (3)将类声明和成员函数的定义分开,放到.h和.cpp中
student.h文件:
```c++
#ifndef STUDENT_H
#define STUDENT_H
#include <string>
class Student {
private:
std::string id; // 学号
std::string name; // 姓名
int score; // 成绩
public:
Student(const std::string& id, const std::string& name, int score);
std::string getId() const;
std::string getName() const;
int getScore() const;
};
#endif // STUDENT_H
```
student.cpp文件:
```c++
#include "student.h"
Student::Student(const std::string& id, const std::string& name, int score) : id(id), name(name), score(score) {}
std::string Student::getId() const {
return id;
}
std::string Student::getName() const {
return name;
}
int Student::getScore() const {
return score;
}
```
main.cpp文件:
```c++
#include <iostream>
#include "student.h"
int main() {
Student students[] = {
Student("001", "Tom", 85),
Student("002", "Jerry", 90),
Student("003", "Mike", 75)
};
for (const auto& student : students) {
int score = student.getScore();
if (score >= 80 && score <= 90) {
std::cout << "id: " << student.getId() << ", name: " << student.getName() << ", score: " << score << std::endl;
}
}
return 0;
}
```
阅读全文
相关推荐
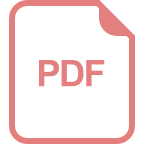
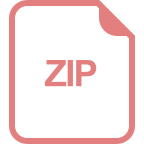
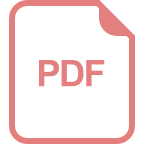
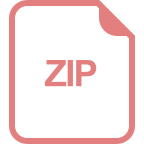
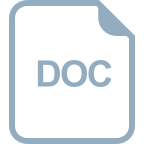
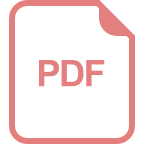
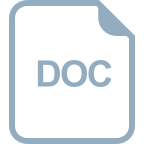
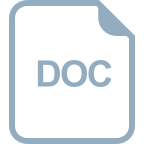
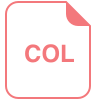
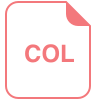
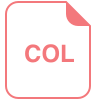
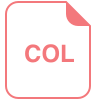



