java@Builder.Default和@Value注入yml配置如何共存
时间: 2023-02-16 21:13:35 浏览: 196
在使用@Builder.Default和@Value注入YML配置时,首先需要在类上添加@Value注解,然后在属性上添加@Builder.Default注解。通过这种方式,在使用建造者模式构建对象时,可以使用YML配置中的默认值,并在需要时进行覆盖。
示例如下:
```
@Value
public class Example {
@Builder.Default
private String name = "defaultValue";
}
```
在YML配置中如下配置
```
example:
name: configValue
```
这样在使用建造者模式创建Example对象时, name属性会优先使用配置文件中的值”configValue”,如果没有配置,则使用默认值”defaultValue”。
相关问题
java项目实现多数据源实例
在Java项目中实现多数据源,可以使用Spring框架的注解和配置来实现。以下是一些实现多数据源的步骤:
1. 配置多个数据源的连接信息,可以在application.properties或application.yml文件中配置多个数据源的连接信息。
2. 在Spring Boot的启动类上添加@MapperScan注解,指定mapper接口所在的包路径。
3. 创建多个DataSource对象,并分别注入到对应的Mapper接口中,可以使用@Qualifier注解指定不同的数据源。
4. 在Mapper接口方法上使用@Mapper注解指定对应的Mapper接口和数据源。
5. 在需要切换数据源的地方,使用@Primary注解指定默认数据源,或者使用@Qualifier注解指定特定的数据源。
6. 在需要动态切换数据源的地方,使用AOP拦截Mapper接口的方法,通过ThreadLocal来保存数据源的信息,并在执行方法前切换数据源。
例如,以下是一个使用Spring Boot和MyBatis实现多数据源的示例代码:
```java
@Configuration
@MapperScan(basePackages = {"com.example.mapper"})
public class DataSourceConfig {
@Bean(name = "dataSource1")
@ConfigurationProperties(prefix = "spring.datasource.db1")
public DataSource dataSource1() {
return DataSourceBuilder.create().build();
}
@Bean(name = "dataSource2")
@ConfigurationProperties(prefix = "spring.datasource.db2")
public DataSource dataSource2() {
return DataSourceBuilder.create().build();
}
@Bean(name = "dynamicDataSource")
@Primary
public DynamicDataSource dataSource(@Qualifier("dataSource1") DataSource dataSource1,
@Qualifier("dataSource2") DataSource dataSource2) {
Map<Object, Object> targetDataSources = new HashMap<>();
targetDataSources.put(DatabaseType.db1, dataSource1);
targetDataSources.put(DatabaseType.db2, dataSource2);
DynamicDataSource dataSource = new DynamicDataSource();
dataSource.setTargetDataSources(targetDataSources);
dataSource.setDefaultTargetDataSource(dataSource1);
return dataSource;
}
}
public enum DatabaseType {
db1, db2;
}
public class DynamicDataSource extends AbstractRoutingDataSource {
@Override
protected Object determineCurrentLookupKey() {
return DatabaseContextHolder.getCurrentDatabaseType();
}
}
public class DatabaseContextHolder {
private static final ThreadLocal<DatabaseType> contextHolder = new ThreadLocal<>();
public static void setCurrentDatabaseType(DatabaseType type) {
contextHolder.set(type);
}
public static DatabaseType getCurrentDatabaseType() {
return contextHolder.get();
}
public static void clearDatabaseType() {
contextHolder.remove();
}
}
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.METHOD})
public @interface DataSource {
DatabaseType value() default DatabaseType.db1;
}
public interface UserMapper {
@DataSource(value = DatabaseType.db1)
List<User> selectAllUsers();
@DataSource(value = DatabaseType.db2)
List<User> selectAllUsersFromDb2();
}
@Aspect
@Component
public class DynamicDataSourceAspect {
@Pointcut("@annotation(com.example.annotation.DataSource)")
public void dataSourcePointCut() {
}
@Around("dataSourcePointCut()")
public Object around(ProceedingJoinPoint point) throws Throwable {
MethodSignature signature = (MethodSignature) point.getSignature();
DataSource dataSource = signature.getMethod().getAnnotation(DataSource.class);
if (dataSource != null) {
DatabaseContextHolder.setCurrentDatabaseType(dataSource.value());
}
try {
return point.proceed();
} finally {
DatabaseContextHolder.clearDatabaseType();
}
}
}
```
以上是一个简单的多数据源的实现,通过注解和AOP实现数据源的动态切换。通过以上实现,可以轻松在Java项目中实现多数据源的功能。
用java写一个mybatis连接多数据库的代码
首先,您需要在项目的配置文件中配置多个数据源。例如,您可以在`application.properties`或`application.yml`文件中配置多个数据源,如下所示:
```
# 数据源1
spring.datasource.first.jdbc-url=jdbc:mysql://localhost:3306/database1
spring.datasource.first.username=root
spring.datasource.first.password=root
# 数据源2
spring.datasource.second.jdbc-url=jdbc:mysql://localhost:3306/database2
spring.datasource.second.username=root
spring.datasource.second.password=root
```
然后,您可以在配置类中配置多个数据源,如下所示:
```java
@Configuration
public class DataSourceConfig {
@Bean
@Primary
@ConfigurationProperties("spring.datasource.first")
public DataSource firstDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
@ConfigurationProperties("spring.datasource.second")
public DataSource secondDataSource() {
return DataSourceBuilder.create().build();
}
}
```
最后,您可以在Mybatis的配置文件中配置多个数据源,如下所示:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/database1"/>
<property name="username" value="root"/>
<property name="password" value="root"/>
</dataSource>
</environment>
<environment id="second">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="url" value="j
阅读全文
相关推荐
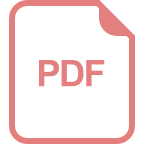
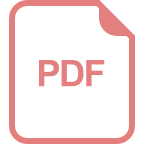
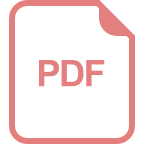
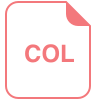
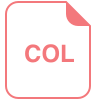
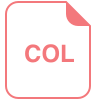
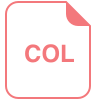
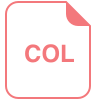
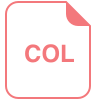
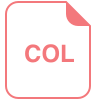
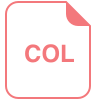
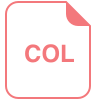
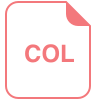
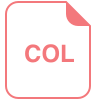
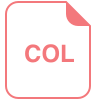

