angular 重新加载当前页面
时间: 2023-09-04 07:04:19 浏览: 79
### 回答1:
在 Angular 中,可以使用 `location.reload()` 方法来重新加载当前页面。你可以在组件的方法中调用这个方法,例如:
```
reloadPage() {
location.reload();
}
```
然后在组件模板中绑定到按钮上,就可以在点击按钮时重新加载页面了。
```
<button (click)="reloadPage()">重新加载页面</button>
```
注意,在使用 `location.reload()` 方法时,整个应用会重新加载,包括组件的初始化和销毁。所以这个方法不是很常用,通常使用其他方法来重新加载数据或更新 UI。
### 回答2:
要在Angular中重新加载当前页面,可以使用`Location`服务的`replaceState`方法。`replaceState`方法会替换当前的浏览历史记录,并且重新加载当前页面。
首先,在组件的构造函数中注入`Location`服务:
```
constructor(private location: Location) { }
```
然后,在需要重新加载页面的方法中,调用`replaceState`方法:
```
reloadPage() {
this.location.replaceState(this.location.path());
window.location.reload();
}
```
`this.location.path()`会返回当前页面的路径,调用`replaceState`方法会将当前页面的浏览历史记录替换为当前页面的路径。然后,使用`window.location.reload()`方法重新加载页面。
另外,也可以使用`Router`服务的`navigateByUrl`方法重新导航到当前页面的URL,达到重新加载页面的效果:
```
constructor(private router: Router) { }
reloadPage() {
const currentUrl = this.router.url;
this.router.navigateByUrl('/', {skipLocationChange: true}).then(() => {
this.router.navigateByUrl(currentUrl);
});
}
```
首先,在组件的构造函数中注入`Router`服务。
然后,在重新加载页面的方法中,先获取当前页面的URL,并使用`navigateByUrl`方法在初始URL('/')上进行导航,并设置`skipLocationChange`选项为`true`,这样可以跳过对浏览历史记录的变更。然后,再通过`navigateByUrl`方法导航到当前页面的URL,达到重新加载页面的效果。
以上是两种在Angular中重新加载当前页面的方法。
### 回答3:
Angular可以通过使用angular-router的`router.navigate()`方法重新加载当前页面。
`router.navigate()`方法可以接受一个路由链接参数,用来指定要导航到的目标链接。如果我们将当前页面的路由链接作为参数传递给`router.navigate()`方法,就可以重新加载当前页面。
以下是一个示例代码:
```typescript
import { Router } from '@angular/router';
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
template: `
<button (click)="reloadPage()">重新加载</button>
`,
})
export class ExampleComponent {
constructor(private router: Router) {}
reloadPage() {
const currentUrl = this.router.url;
this.router.navigate([currentUrl]);
}
}
```
在上面的代码中,我们通过将`this.router.url`作为参数传递给`router.navigate()`方法,来重新加载当前页面。
当点击"重新加载"按钮时,应用程序将重新导航到当前页面的路由链接,从而重新加载该页面。
请注意,这种重新加载页面的方法只适用于Angular的单页面应用程序,如果我们尝试在多个页面之间进行页面重新加载,将会导致整个应用程序重新加载。
相关推荐
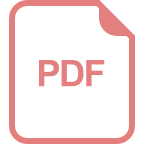
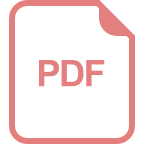














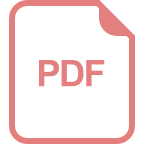