vue3 ts 实现导航锚点
时间: 2023-08-29 13:13:24 浏览: 60
实现导航锚点需要用到Vue3的ref、computed、watchEffect、scrollTo等函数,以及HTML的锚点标签<a>。
1. 在Vue3的setup函数中,定义一个ref变量来存储当前选中的锚点的id值。
```typescript
import { ref } from 'vue'
export default {
setup() {
const currentAnchor = ref('')
// ...
}
}
```
2. 使用computed函数来获取页面中所有的锚点元素,并将它们的id值存储到一个数组中。
```typescript
import { ref, computed, onMounted } from 'vue'
export default {
setup() {
const currentAnchor = ref('')
const anchorList = computed(() => {
return Array.from(document.querySelectorAll('a[href^="#"]')).map(item => {
return item.getAttribute('href').slice(1)
})
})
// ...
}
}
```
3. 使用watchEffect函数来监听页面滚动事件,并根据当前滚动位置来更新当前选中的锚点的id值。
```typescript
import { ref, computed, onMounted, watchEffect } from 'vue'
export default {
setup() {
const currentAnchor = ref('')
const anchorList = computed(() => {
return Array.from(document.querySelectorAll('a[href^="#"]')).map(item => {
return item.getAttribute('href').slice(1)
})
})
onMounted(() => {
watchEffect(() => {
const scrollTop = document.documentElement.scrollTop || document.body.scrollTop
let selectedAnchor = ''
for (let i = 0; i < anchorList.value.length; i++) {
const anchorElement = document.getElementById(anchorList.value[i])
if (anchorElement && anchorElement.offsetTop <= scrollTop + 100) {
selectedAnchor = anchorList.value[i]
}
}
currentAnchor.value = selectedAnchor
})
})
// ...
}
}
```
4. 在模板中通过v-for指令来循环渲染所有的锚点,并使用class绑定来动态设置当前选中的锚点的样式。
```html
<template>
<div>
<ul>
<li v-for="anchor in anchorList" :key="anchor">
<a :href="'#' + anchor" :class="{ active: currentAnchor === anchor }">{{ anchor }}</a>
</li>
</ul>
<div id="anchor1">锚点1</div>
<div id="anchor2">锚点2</div>
<div id="anchor3">锚点3</div>
</div>
</template>
<script>
import { ref, computed, onMounted, watchEffect } from 'vue'
export default {
setup() {
const currentAnchor = ref('')
const anchorList = computed(() => {
return Array.from(document.querySelectorAll('a[href^="#"]')).map(item => {
return item.getAttribute('href').slice(1)
})
})
onMounted(() => {
watchEffect(() => {
const scrollTop = document.documentElement.scrollTop || document.body.scrollTop
let selectedAnchor = ''
for (let i = 0; i < anchorList.value.length; i++) {
const anchorElement = document.getElementById(anchorList.value[i])
if (anchorElement && anchorElement.offsetTop <= scrollTop + 100) {
selectedAnchor = anchorList.value[i]
}
}
currentAnchor.value = selectedAnchor
})
})
return {
currentAnchor,
anchorList
}
}
}
</script>
<style>
.active {
color: red;
}
</style>
```
以上代码就是一个简单的Vue3+TypeScript实现导航锚点的示例。
相关推荐
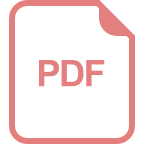














