用vue3写一个功能:点击登录按钮,自动校验账号框和密码框,为空则给出提示“账号或密码不能为空!”,并判断账号(11位手机号)和密码(8-20位数字和字母)是否正确,,不正确则提示“用户名和密码错误!”要求美观一点
时间: 2023-12-18 19:02:24 浏览: 71
以下是一个简单的示例代码,使用了Vue3的Composition API来实现:
```html
<template>
<div class="login-form">
<h2>登录</h2>
<form @submit.prevent="submitForm">
<div class="form-group">
<label for="username">账号</label>
<input type="text" id="username" v-model="username" placeholder="请输入11位手机号码" />
</div>
<div class="form-group">
<label for="password">密码</label>
<input type="password" id="password" v-model="password" placeholder="请输入8-20位数字和字母" />
</div>
<div class="form-group">
<button type="submit">登录</button>
</div>
</form>
<div v-if="errorMessage" class="error-message">{{ errorMessage }}</div>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const username = ref('');
const password = ref('');
const errorMessage = ref('');
const submitForm = () => {
if (!username.value || !password.value) {
errorMessage.value = '账号或密码不能为空!';
return;
}
const phoneRegex = /^1\d{10}$/;
const passwordRegex = /^[a-zA-Z0-9]{8,20}$/;
if (!phoneRegex.test(username.value) || !passwordRegex.test(password.value)) {
errorMessage.value = '用户名和密码错误!';
return;
}
// TODO: 发送登录请求
errorMessage.value = '';
};
return {
username,
password,
errorMessage,
submitForm,
};
},
};
</script>
<style scoped>
.login-form {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3);
}
.form-group {
margin-bottom: 10px;
}
label {
display: block;
margin-bottom: 5px;
}
input[type="text"],
input[type="password"] {
width: 100%;
padding: 8px;
border: 1px solid #ccc;
border-radius: 3px;
}
button[type="submit"] {
background-color: #4CAF50;
color: #fff;
padding: 8px 16px;
border: none;
border-radius: 3px;
cursor: pointer;
transition: background-color 0.2s;
}
button[type="submit"]:hover {
background-color: #3e8e41;
}
.error-message {
color: red;
margin-top: 10px;
}
</style>
```
在这个示例代码中,我们使用了Vue3的Composition API来编写组件逻辑。`ref`函数用于创建响应式数据,我们使用它来创建`username`、`password`和`errorMessage`变量。
我们在模板中使用了`v-model`指令来双向绑定输入框的值,使用`@submit`事件监听表单提交事件,调用`submitForm`方法来处理登录逻辑。
`submitForm`方法首先检查账号和密码是否为空,如果为空则设置`errorMessage`为相应的提示信息并返回。然后使用正则表达式检查账号和密码是否符合格式要求,如果不符合则同样设置`errorMessage`为相应的提示信息并返回。
最后,如果账号和密码都符合要求,我们可以发送登录请求,并清空`errorMessage`以便下次使用。如果你需要根据具体的业务逻辑来处理登录请求,可以在TODO注释下面编写相应的代码。
最后,我们使用了一些简单的CSS样式来美化表单,包括设置表单宽度、边框、圆角、阴影等等。同时,我们也为错误提示信息设置了一个红色颜色。
相关推荐
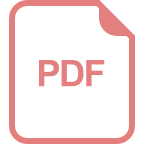
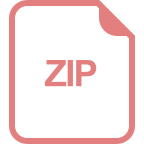










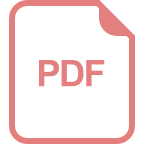
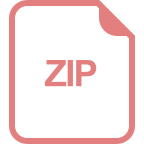