用c语言实现响应比高者优先算法的作业调度
时间: 2023-09-22 22:15:35 浏览: 49
好的,下面是用C语言实现响应比高者优先算法的作业调度的代码:
```c
#include <stdio.h>
#define MAX_SIZE 100
int main()
{
int n, i, j;
int process[MAX_SIZE], burst_time[MAX_SIZE], wait_time[MAX_SIZE], turnaround_time[MAX_SIZE], response_ratio[MAX_SIZE], completed[MAX_SIZE];
float avg_waiting_time = 0, avg_turnaround_time = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time of each process: \n");
for (i = 0; i < n; i++)
{
printf("Process[%d]: ", i + 1);
scanf("%d", &burst_time[i]);
process[i] = i + 1;
completed[i] = 0;
}
int time = 0, shortest_job_index = 0, complete_count = 0;
while (complete_count != n)
{
for (i = 0; i < n; i++)
{
if (completed[i] == 0 && burst_time[i] < burst_time[shortest_job_index])
{
shortest_job_index = i;
}
if (completed[i] == 0)
{
response_ratio[i] = 1 + (time - wait_time[i]) / burst_time[i];
}
}
wait_time[shortest_job_index] = time;
time += burst_time[shortest_job_index];
turnaround_time[shortest_job_index] = time;
completed[shortest_job_index] = 1;
complete_count++;
avg_waiting_time += wait_time[shortest_job_index];
avg_turnaround_time += turnaround_time[shortest_job_index];
for (i = 0; i < n; i++)
{
if (completed[i] == 0)
{
response_ratio[i] = 1 + (time - wait_time[i]) / burst_time[i];
}
}
shortest_job_index = 0;
for (i = 0; i < n; i++)
{
if (completed[i] == 0 && response_ratio[i] > response_ratio[shortest_job_index])
{
shortest_job_index = i;
}
}
}
avg_waiting_time /= n;
avg_turnaround_time /= n;
printf("\nProcess\tBurst Time\tWaiting Time\tTurnaround Time\n");
for (i = 0; i < n; i++)
{
printf("P[%d]\t\t%d\t\t%d\t\t%d\n", process[i], burst_time[i], wait_time[i], turnaround_time[i]);
}
printf("\nAverage waiting time: %.2f", avg_waiting_time);
printf("\nAverage turnaround time: %.2f", avg_turnaround_time);
return 0;
}
```
该算法首先会要求用户输入进程数和每个进程的处理时间,然后计算每个进程的响应比,选择响应比最高的进程进行处理,直到所有进程都处理完毕为止。输出结果包括每个进程的处理时间、等待时间和周转时间,以及平均等待时间和平均周转时间。
相关推荐
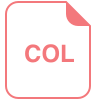
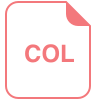
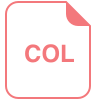
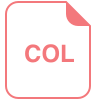
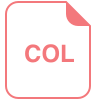









