用C++使用类模板设计一个循环队列
时间: 2023-11-27 08:52:01 浏览: 75
好的,下面是一个使用类模板实现循环队列的示例代码:
```cpp
#include <iostream>
using namespace std;
template <typename T>
class Queue {
private:
T *data; // 存储数据的数组
int head; // 队头指针
int tail; // 队尾指针
int size; // 队列大小
public:
Queue(int s = 10) : data(new T[s]), head(0), tail(0), size(s) {}
~Queue() { delete[] data; }
bool empty() const { return head == tail; } // 判断队列是否为空
bool full() const { return (tail + 1) % size == head; } // 判断队列是否已满
void clear() { head = tail = 0; } // 清空队列
int length() const { return (tail - head + size) % size; } // 返回队列长度
bool enqueue(const T &value) { // 入队操作
if (full()) {
cout << "队列已满,无法入队!" << endl;
return false;
}
data[tail] = value;
tail = (tail + 1) % size;
return true;
}
bool dequeue(T &value) { // 出队操作
if (empty()) {
cout << "队列为空,无法出队!" << endl;
return false;
}
value = data[head];
head = (head + 1) % size;
return true;
}
void print() const { // 输出队列中的所有元素
if (empty()) {
cout << "队列为空!" << endl;
return;
}
int i = head;
while (i != tail) {
cout << data[i] << " ";
i = (i + 1) % size;
}
cout << endl;
}
};
int main() {
Queue<int> q(5); // 创建一个能存储5个int类型元素的队列
q.enqueue(1);
q.enqueue(2);
q.enqueue(3);
q.print(); // 输出:1 2 3
int value;
q.dequeue(value);
cout << "出队元素:" << value << endl; // 输出:出队元素:1
q.enqueue(4);
q.enqueue(5);
q.print(); // 输出:2 3 4 5
q.enqueue(6); // 输出:队列已满,无法入队!
q.clear();
q.print(); // 输出:队列为空!
return 0;
}
```
在上面的示例代码中,我们使用了模板类 `Queue` 来表示一个循环队列。它的私有成员包括:
- `data`:一个指向存储队列元素的数组的指针;
- `head`:一个指向队头元素的指针;
- `tail`:一个指向队尾元素的指针;
- `size`:队列的大小。
其中,`head` 指向队头元素,`tail` 指向队尾元素的下一个位置。为了方便计算队列长度,我们使用了取模运算。
`Queue` 类提供了以下公有成员函数:
- `Queue(int s = 10)`:构造函数,创建一个大小为 `s` 的循环队列。如果不传入参数,则默认创建一个大小为10的队列;
- `~Queue()`:析构函数,释放存储队列元素的数组占用的内存;
- `bool empty() const`:判断队列是否为空,如果队头指针等于队尾指针,说明队列为空;
- `bool full() const`:判断队列是否已满,如果队尾指针的下一个位置等于队头指针,说明队列已满;
- `void clear()`:清空队列,将队头指针和队尾指针都置为0;
- `int length() const`:返回队列中元素的个数;
- `bool enqueue(const T &value)`:将元素 `value` 入队。如果队列已满,则入队失败;
- `bool dequeue(T &value)`:将队头元素出队,并将其保存到 `value` 中。如果队列为空,则出队失败;
- `void print() const`:输出队列中的所有元素。
在 `main` 函数中,我们通过调用 `enqueue` 和 `dequeue` 函数来操作队列,并使用 `print` 函数输出队列中的元素。
阅读全文
相关推荐



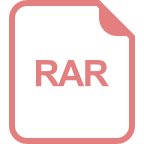











