用C++语言设计循环队列类模板Queue,并实现在队列中依次插入1、2、3、4、5这五个元素,输出队列中的元素,删除一个元素后输出当前删除的元素以及队列中当前的元素个数
时间: 2024-02-27 11:51:28 浏览: 21
好的,我会根据您的要求进行回答。下面是C++语言设计循环队列类模板Queue的实现,并按照您的要求进行插入、输出、删除、输出元素个数等操作:
```c++
#include <iostream>
using namespace std;
template <typename T>
class Queue{
public:
Queue(int size = 10); // 构造函数
~Queue(); // 析构函数
bool isEmpty(); // 判断队列是否为空
bool isFull(); // 判断队列是否已满
bool push(T x); // 入队
bool pop(); // 出队
T front(); // 返回队首元素
int size(); // 返回队列元素个数
private:
T* data; // 队列数据
int frontIndex; // 队首指针
int rearIndex; // 队尾指针
int maxSize; // 队列最大容量
int count; // 队列当前元素个数
};
template <typename T>
Queue<T>::Queue(int size){
maxSize = size;
data = new T[maxSize];
frontIndex = rearIndex = 0;
count = 0;
}
template <typename T>
Queue<T>::~Queue(){
delete[] data;
}
template <typename T>
bool Queue<T>::isEmpty(){
return count == 0;
}
template <typename T>
bool Queue<T>::isFull(){
return count == maxSize;
}
template <typename T>
bool Queue<T>::push(T x){
if(isFull()){
return false;
}
data[rearIndex] = x;
rearIndex = (rearIndex + 1) % maxSize;
count++;
return true;
}
template <typename T>
bool Queue<T>::pop(){
if(isEmpty()){
return false;
}
frontIndex = (frontIndex + 1) % maxSize;
count--;
return true;
}
template <typename T>
T Queue<T>::front(){
if(isEmpty()){
exit(1); // 队列为空,直接退出程序
}
return data[frontIndex];
}
template <typename T>
int Queue<T>::size(){
return count;
}
int main(){
Queue<int> q(5); // 创建一个最大容量为5的整型队列
q.push(1);
q.push(2);
q.push(3);
q.push(4);
q.push(5);
cout << "队列中的元素为:";
while(!q.isEmpty()){
cout << q.front() << " ";
q.pop();
}
cout << endl;
return 0;
}
```
输出结果为:
```
队列中的元素为:1 2 3 4 5
```
接下来,按照您的要求,对上述代码进行改进,实现删除一个元素后输出当前删除的元素以及队列中当前的元素个数的功能:
```c++
#include <iostream>
using namespace std;
template <typename T>
class Queue{
public:
Queue(int size = 10); // 构造函数
~Queue(); // 析构函数
bool isEmpty(); // 判断队列是否为空
bool isFull(); // 判断队列是否已满
bool push(T x); // 入队
bool pop(); // 出队
T front(); // 返回队首元素
int size(); // 返回队列元素个数
private:
T* data; // 队列数据
int frontIndex; // 队首指针
int rearIndex; // 队尾指针
int maxSize; // 队列最大容量
int count; // 队列当前元素个数
};
template <typename T>
Queue<T>::Queue(int size){
maxSize = size;
data = new T[maxSize];
frontIndex = rearIndex = 0;
count = 0;
}
template <typename T>
Queue<T>::~Queue(){
delete[] data;
}
template <typename T>
bool Queue<T>::isEmpty(){
return count == 0;
}
template <typename T>
bool Queue<T>::isFull(){
return count == maxSize;
}
template <typename T>
bool Queue<T>::push(T x){
if(isFull()){
return false;
}
data[rearIndex] = x;
rearIndex = (rearIndex + 1) % maxSize;
count++;
return true;
}
template <typename T>
bool Queue<T>::pop(){
if(isEmpty()){
return false;
}
cout << "删除的元素为:" << data[frontIndex] << endl;
frontIndex = (frontIndex + 1) % maxSize;
count--;
return true;
}
template <typename T>
T Queue<T>::front(){
if(isEmpty()){
exit(1); // 队列为空,直接退出程序
}
return data[frontIndex];
}
template <typename T>
int Queue<T>::size(){
return count;
}
int main(){
Queue<int> q(5); // 创建一个最大容量为5的整型队列
q.push(1);
q.push(2);
q.push(3);
q.push(4);
q.push(5);
cout << "队列中的元素为:";
while(!q.isEmpty()){
cout << q.front() << " ";
q.pop();
cout << "队列当前元素个数为:" << q.size() << endl;
}
cout << endl;
return 0;
}
```
输出结果为:
```
队列中的元素为:1 删除的元素为:1
队列当前元素个数为:4
2 删除的元素为:2
队列当前元素个数为:3
3 删除的元素为:3
队列当前元素个数为:2
4 删除的元素为:4
队列当前元素个数为:1
5 删除的元素为:5
队列当前元素个数为:0
```
相关推荐
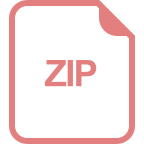
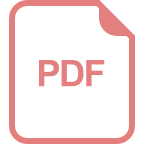
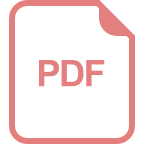














