c++ 设计一个选择式菜单1. 实现顺序循环或链式队列的进队列、出队列、判断队列空否、显示队列中全部元素的运算
时间: 2024-01-27 11:05:27 浏览: 32
以下是基于顺序循环队列的实现:
```c++
#include<iostream>
using namespace std;
const int MAXSIZE = 100; //队列最大容量
template<typename T>
class Queue {
private:
T data[MAXSIZE]; //队列数组
int front; //队头指针
int rear; //队尾指针
public:
Queue() { //构造函数
front = rear = 0;
}
bool isEmpty() { //判断队列是否为空
return front == rear;
}
bool isFull() { //判断队列是否满
return (rear + 1) % MAXSIZE == front;
}
void enQueue(T x) { //进队列
if (isFull()) {
cout << "Queue is full." << endl;
return;
}
data[rear] = x;
rear = (rear + 1) % MAXSIZE;
}
void deQueue() { //出队列
if (isEmpty()) {
cout << "Queue is empty." << endl;
return;
}
front = (front + 1) % MAXSIZE;
}
void display() { //显示队列中全部元素
if (isEmpty()) {
cout << "Queue is empty." << endl;
return;
}
cout << "Elements in the queue: ";
int i = front;
while (i != rear) {
cout << data[i] << " ";
i = (i + 1) % MAXSIZE;
}
cout << endl;
}
};
int main() {
Queue<int> q;
int choice, x;
while (1) { //循环显示菜单
cout << "1. Enqueue" << endl;
cout << "2. Dequeue" << endl;
cout << "3. Check if queue is empty" << endl;
cout << "4. Display all elements in the queue" << endl;
cout << "5. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "Enter the element to enqueue: ";
cin >> x;
q.enQueue(x);
break;
case 2:
q.deQueue();
break;
case 3:
if (q.isEmpty())
cout << "Queue is empty." << endl;
else
cout << "Queue is not empty." << endl;
break;
case 4:
q.display();
break;
case 5:
return 0;
default:
cout << "Invalid choice." << endl;
break;
}
}
return 0;
}
```
需要注意的是,在顺序循环队列中,需要判断队列是否满时,不能直接将队尾指针加1后和队头指针比较,因为队尾指针可能已经到达队列最后一个位置,此时需要将其循环回到队列开头。因此,判断队列是否满的公式为 `(rear + 1) % MAXSIZE == front`。出队列时同理,需要将队头指针加1后循环回到队列开头。
相关推荐
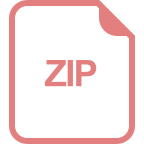
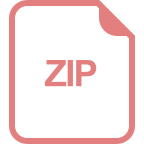
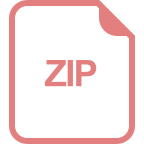
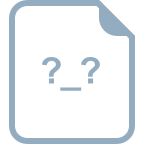
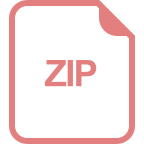
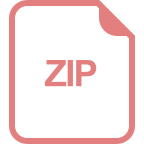
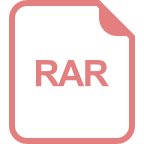
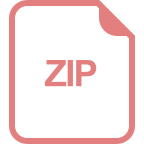
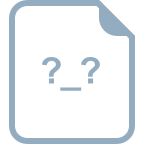
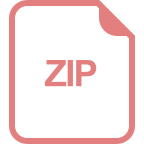
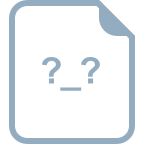
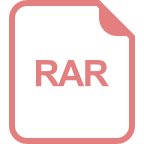
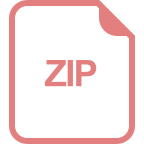
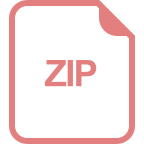
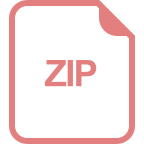