利用循环队列的基本运算和《教程》中例3.13求循环队列元素个数的算法,用c++生成代码
时间: 2023-07-24 14:42:00 浏览: 46
好的,以下是基于循环队列的基本运算和例3.13求循环队列元素个数的算法的C++代码:
```c++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 队列最大长度
template<typename T>
class CircularQueue {
private:
T queue[MAXSIZE]; // 队列数组
int front, rear; // 队头、队尾指针
public:
CircularQueue() { // 初始化队列
front = rear = 0;
}
bool isEmpty() { // 判断队列是否为空
return front == rear;
}
bool isFull() { // 判断队列是否已满
return (rear + 1) % MAXSIZE == front;
}
int size() { // 求队列元素个数
return (rear - front + MAXSIZE) % MAXSIZE;
}
void enqueue(T data) { // 入队
if (isFull()) {
cout << "Queue is full!" << endl;
return;
}
queue[rear] = data;
rear = (rear + 1) % MAXSIZE;
}
T dequeue() { // 出队
if (isEmpty()) {
cout << "Queue is empty!" << endl;
exit(1);
}
T data = queue[front];
front = (front + 1) % MAXSIZE;
return data;
}
};
int main() {
// 创建一个循环队列
CircularQueue<int> queue;
// 入队
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
// 求队列元素个数并输出
cout << "Queue size: " << queue.size() << endl;
// 出队
queue.dequeue();
// 求队列元素个数并输出
cout << "Queue size: " << queue.size() << endl;
return 0;
}
```
以上代码中,我们定义了一个 `CircularQueue` 类,包含了循环队列的基本运算,如入队、出队、判断队列是否为空、判断队列是否已满等。同时,我们还定义了一个 `size` 方法,用于求循环队列的元素个数,其实现与例3.13中的算法一致。在 `main` 函数中,我们创建了一个 `CircularQueue` 对象,并对其进行了一些操作,以检验其正确性。
相关推荐
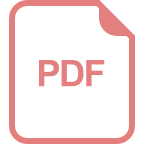
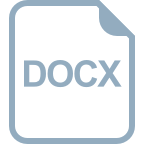














