const express = require('express'); const mysql = require('mysql'); const app = express(); const port = process.env.PORT || 3002; app.use(express.static('public')); app.get('/data', (req, res) => { // 创建MySQL连接 const connection = mysql.createConnection({ host: 'localhost', user: 'root', port:'3306', password: '123456', database: 'school' }); // 连接到MySQL数据库 connection.connect((err) => { if (err) throw err; // 从数据库中获取数据 connection.query('SELECT * FROM ahp', (err, data) => { if (err) throw err; // 将数据发送给客户端 res.send(data); connection.end(); }); }); }); app.listen(port, () => { console.log(`Server listening at http://localhost:${port}`); });打开http://localhost:3000网页显示Cannot GET /
时间: 2024-03-09 14:45:57 浏览: 20
这段代码中,你将 express 应用程序监听的端口设置为了 3002,但是你在打开网页时使用了 3000 端口。应该将打开网页时的端口改为 3002,即 http://localhost:3002。
另外,应该使用 `app.get('/'` 路径,而不是 `app.get('/data'`,因为你的网页的根路径是 `/`。如果想使用 `/data` 路径,可以将网页的链接改为 http://localhost:3002/data。
更改后的代码如下:
```
const express = require('express');
const mysql = require('mysql');
const app = express();
const port = process.env.PORT || 3002;
app.use(express.static('public'));
app.get('/', (req, res) => {
// 创建MySQL连接
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
port: '3306',
password: '123456',
database: 'school'
});
// 连接到MySQL数据库
connection.connect((err) => {
if (err) throw err;
// 从数据库中获取数据
connection.query('SELECT * FROM ahp', (err, data) => {
if (err) throw err;
// 将数据发送给客户端
res.send(data);
connection.end();
});
});
});
app.listen(port, () => {
console.log(`Server listening at http://localhost:${port}`);
});
```
相关推荐
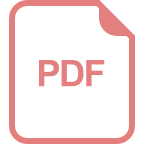
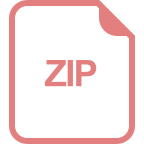
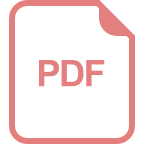











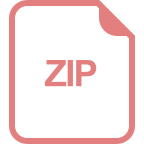
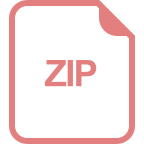
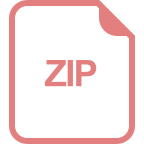
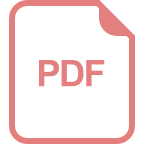