c程序设计1)linux坏境实现对write命令的中文支持 (2)基于Linux文件系统的功能,实现不同用户间的通信 (3)支持管道、重定向
时间: 2024-02-11 08:09:03 浏览: 22
1)要在Linux环境中实现对write命令的中文支持,可以使用以下代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <wchar.h>
#include <locale.h>
int main() {
setlocale(LC_ALL, ""); // 设置本地化环境
wchar_t str[] = L"你好,世界!"; // 宽字符类型字符串
write(1, str, wcslen(str) * sizeof(wchar_t)); // 输出到标准输出
return 0;
}
```
2)要基于Linux文件系统实现不同用户间的通信,可以使用以下代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#define BUFSIZE 1024
int main() {
char buf[BUFSIZE];
int fd; // 文件描述符
pid_t pid; // 进程ID
// 创建命名管道
if (mkfifo("myfifo", 0666) < 0) {
perror("mkfifo");
exit(1);
}
// 创建子进程
pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
} else if (pid == 0) { // 子进程
fd = open("myfifo", O_WRONLY); // 只写模式打开管道
if (fd < 0) {
perror("open");
exit(1);
}
write(fd, "Hello, parent!", strlen("Hello, parent!"));
close(fd);
exit(0);
} else { // 父进程
fd = open("myfifo", O_RDONLY); // 只读模式打开管道
if (fd < 0) {
perror("open");
exit(1);
}
read(fd, buf, BUFSIZE);
printf("Received message: %s\n", buf);
close(fd);
wait(); // 等待子进程结束
exit(0);
}
return 0;
}
```
3)要支持管道和重定向,可以使用以下代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main() {
int fd[2];
pid_t pid;
// 创建管道
if (pipe(fd) < 0) {
perror("pipe");
exit(1);
}
// 创建子进程
pid = fork();
if (pid < 0) {
perror("fork");
exit(1);
} else if (pid == 0) { // 子进程
close(fd[0]); // 关闭读端
dup2(fd[1], STDOUT_FILENO); // 标准输出重定向到管道写端
execlp("ls", "ls", "-al", NULL); // 执行ls命令
exit(0);
} else { // 父进程
close(fd[1]); // 关闭写端
dup2(fd[0], STDIN_FILENO); // 标准输入重定向到管道读端
execlp("wc", "wc", "-l", NULL); // 执行wc命令
wait(); // 等待子进程结束
exit(0);
}
return 0;
}
```
相关推荐
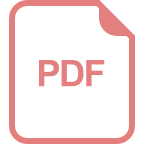
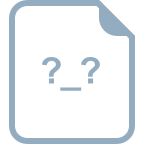
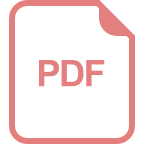














