使用java设计一个类Shape(图形)包含求面积和周长的area()方法和perimeter()方法以及设置颜色的方法SetColor(),并设计其子类Circle(圆形)类、Rectangle(矩形)类,并分别实现相应的求面积和求周长的方法。每个类都要覆盖toString方法。
时间: 2024-03-08 17:46:04 浏览: 48
好的,根据您的需求,我给您提供一份Java代码,如下所示:
```
public abstract class Shape {
protected String color;
public Shape(String color) {
this.color = color;
}
public abstract double area();
public abstract double perimeter();
public void setColor(String color) {
this.color = color;
}
@Override
public String toString() {
return "Shape(color=" + color + ")";
}
}
public class Circle extends Shape {
protected double radius;
public Circle(String color, double radius) {
super(color);
this.radius = radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
@Override
public double perimeter() {
return 2 * Math.PI * radius;
}
@Override
public String toString() {
return "Circle(color=" + color + ", radius=" + radius + ")";
}
}
public class Rectangle extends Shape {
protected double width;
protected double height;
public Rectangle(String color, double width, double height) {
super(color);
this.width = width;
this.height = height;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
@Override
public double area() {
return width * height;
}
@Override
public double perimeter() {
return 2 * (width + height);
}
@Override
public String toString() {
return "Rectangle(color=" + color + ", width=" + width + ", height=" + height + ")";
}
}
```
在上面的代码中,我们定义了一个抽象类 Shape,其中包含了求面积、周长和设置颜色的方法。然后我们又定义了两个子类 Circle 和 Rectangle,分别实现了 Shape 类的抽象方法,并覆盖了 toString 方法。这样,我们就可以方便地创建不同的图形对象,并且获取它们的面积、周长和颜色信息了。
相关推荐
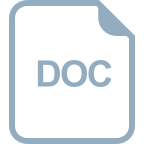















