如何利用QT序列化实现文件加密
时间: 2023-10-19 22:07:30 浏览: 144
利用QT序列化实现文件加密的基本思路是:将需要加密的文件先进行序列化,然后对序列化后的数据进行加密,最后将加密后的数据写入文件。反之,解密则是先读取加密后的文件,将数据进行解密,然后进行反序列化,得到原始数据。
以下是一个简单的加密和解密示例代码,供参考:
```cpp
// 加密函数
void encryptFile(const QString& originalFilePath, const QString& encryptedFilePath, const QString& password)
{
QFile originalFile(originalFilePath);
QFile encryptedFile(encryptedFilePath);
if (!originalFile.open(QIODevice::ReadOnly))
return;
if (!encryptedFile.open(QIODevice::WriteOnly))
return;
// 创建数据流对象
QDataStream in(&originalFile);
QDataStream out(&encryptedFile);
// 设置数据流版本
in.setVersion(QDataStream::Qt_5_15);
out.setVersion(QDataStream::Qt_5_15);
// 读取原始数据并进行加密
QByteArray originalData = originalFile.readAll();
QByteArray encryptedData = QByteArray::fromRawData(originalData.constData(), originalData.size());
encryptedData = encryptedData.toBase64().toLatin1();
int keyIndex = 0;
for (int i = 0; i < encryptedData.size(); i++)
{
encryptedData[i] = encryptedData[i] ^ password[keyIndex].toLatin1();
keyIndex = (keyIndex + 1) % password.size();
}
// 序列化并写入加密后的数据
out << encryptedData;
originalFile.close();
encryptedFile.close();
}
// 解密函数
void decryptFile(const QString& encryptedFilePath, const QString& decryptedFilePath, const QString& password)
{
QFile encryptedFile(encryptedFilePath);
QFile decryptedFile(decryptedFilePath);
if (!encryptedFile.open(QIODevice::ReadOnly))
return;
if (!decryptedFile.open(QIODevice::WriteOnly))
return;
// 创建数据流对象
QDataStream in(&encryptedFile);
QDataStream out(&decryptedFile);
// 设置数据流版本
in.setVersion(QDataStream::Qt_5_15);
out.setVersion(QDataStream::Qt_5_15);
// 读取加密后的数据并进行解密
QByteArray encryptedData;
in >> encryptedData;
int keyIndex = 0;
for (int i = 0; i < encryptedData.size(); i++)
{
encryptedData[i] = encryptedData[i] ^ password[keyIndex].toLatin1();
keyIndex = (keyIndex + 1) % password.size();
}
encryptedData = QByteArray::fromBase64(encryptedData);
// 反序列化并写入解密后的数据
decryptedFile.write(encryptedData);
encryptedFile.close();
decryptedFile.close();
}
```
在上述示例代码中,使用了XOR加密算法对数据进行加密和解密。加密过程将原始数据进行序列化,然后将序列化后的数据进行加密,并写入加密后的文件。解密过程则是读取加密后的文件,将加密后的数据进行解密,然后进行反序列化,得到原始数据,并写入解密后的文件。需要注意的是,加密和解密过程中使用的密码应该保密并足够强大,以免被攻击者破解。
阅读全文
相关推荐


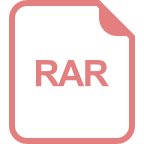


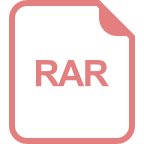

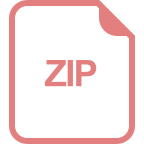





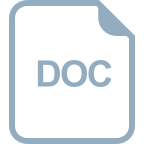



