1.完成的功能包括如下几点: ①编程实现建立一棵二叉树,然后对其进行先序、中序和后序遍历。 分析:将要输入的二叉树按照其对应的完全二叉树的顺序输入,若当前位置不存在结点则输入@ ②显示二叉树 ③求二叉树的高度及二叉树的叶子个数等等 ④在主函数中设计一个简单的菜单,分别调试上述算法 C语言数据结构主函数代码
时间: 2024-02-18 15:05:38 浏览: 72
以下是一个简单的C语言数据结构程序,实现了上述要求的功能:
```
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
char val;
struct TreeNode *left;
struct TreeNode *right;
};
// 先序遍历
void preorder(struct TreeNode *root) {
if (root) {
printf("%c ", root->val);
preorder(root->left);
preorder(root->right);
}
}
// 中序遍历
void inorder(struct TreeNode *root) {
if (root) {
inorder(root->left);
printf("%c ", root->val);
inorder(root->right);
}
}
// 后序遍历
void postorder(struct TreeNode *root) {
if (root) {
postorder(root->left);
postorder(root->right);
printf("%c ", root->val);
}
}
// 创建二叉树
struct TreeNode* createTree() {
struct TreeNode *root;
char val;
scanf("%c", &val);
if (val == '@') {
root = NULL;
} else {
root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = val;
root->left = createTree();
root->right = createTree();
}
return root;
}
// 显示二叉树
void printTree(struct TreeNode *root, int depth) {
if (root) {
int i;
for (i = 0; i < depth; i++) {
printf(" ");
}
printf("%c\n", root->val);
printTree(root->left, depth + 1);
printTree(root->right, depth + 1);
}
}
// 计算二叉树高度
int getHeight(struct TreeNode *root) {
if (root == NULL) {
return 0;
} else {
int leftHeight = getHeight(root->left);
int rightHeight = getHeight(root->right);
return leftHeight > rightHeight ? leftHeight + 1 : rightHeight + 1;
}
}
// 计算二叉树叶子节点个数
int getLeafCount(struct TreeNode *root) {
if (root == NULL) {
return 0;
} else if (root->left == NULL && root->right == NULL) {
return 1;
} else {
return getLeafCount(root->left) + getLeafCount(root->right);
}
}
int main() {
struct TreeNode *root;
int choice;
printf("请输入二叉树的结点,空结点用@表示:\n");
root = createTree();
while (1) {
printf("\n");
printf("1. 先序遍历\n");
printf("2. 中序遍历\n");
printf("3. 后序遍历\n");
printf("4. 显示二叉树\n");
printf("5. 计算二叉树高度\n");
printf("6. 计算二叉树叶子节点个数\n");
printf("7. 退出\n");
printf("请输入您的选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("先序遍历结果为:");
preorder(root);
printf("\n");
break;
case 2:
printf("中序遍历结果为:");
inorder(root);
printf("\n");
break;
case 3:
printf("后序遍历结果为:");
postorder(root);
printf("\n");
break;
case 4:
printf("二叉树如下:\n");
printTree(root, 0);
break;
case 5:
printf("二叉树高度为:%d\n", getHeight(root));
break;
case 6:
printf("二叉树叶子节点个数为:%d\n", getLeafCount(root));
break;
case 7:
exit(0);
default:
printf("输入有误,请重新输入。\n");
break;
}
}
return 0;
}
```
阅读全文
相关推荐







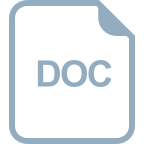









