linux使用udev检测usb热插拔的代码例子, 要创建一个工作线程
时间: 2024-04-29 11:22:09 浏览: 239
下面是一个使用udev检测USB设备热插拔并创建工作线程的代码例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <libudev.h>
void *worker_thread(void *arg) {
printf("Worker thread started\n");
while (1) {
printf("Working...\n");
sleep(1);
}
return NULL;
}
int main() {
struct udev *udev;
struct udev_monitor *mon;
int fd;
fd_set fds;
pthread_t thread;
// 创建udev实例
udev = udev_new();
if (!udev) {
fprintf(stderr, "Failed to create udev instance\n");
return 1;
}
// 创建监视器并设置监听事件类型
mon = udev_monitor_new_from_netlink(udev, "udev");
if (!mon) {
fprintf(stderr, "Failed to create udev monitor\n");
udev_unref(udev);
return 1;
}
udev_monitor_filter_add_match_subsystem_devtype(mon, "usb", "usb_device");
// 开始监听
if (udev_monitor_enable_receiving(mon)) {
fprintf(stderr, "Failed to enable udev monitor receiving\n");
udev_monitor_unref(mon);
udev_unref(udev);
return 1;
}
fd = udev_monitor_get_fd(mon);
// 创建工作线程
if (pthread_create(&thread, NULL, worker_thread, NULL)) {
fprintf(stderr, "Failed to create worker thread\n");
udev_monitor_unref(mon);
udev_unref(udev);
return 1;
}
// 监听事件并处理
while (1) {
FD_ZERO(&fds);
FD_SET(fd, &fds);
if (select(fd + 1, &fds, NULL, NULL, NULL) <= 0)
continue;
if (FD_ISSET(fd, &fds)) {
struct udev_device *dev;
dev = udev_monitor_receive_device(mon);
if (dev) {
printf("USB device %s %s\n", udev_device_get_action(dev), udev_device_get_sysname(dev));
udev_device_unref(dev);
}
}
}
// 清理
pthread_join(thread, NULL);
udev_monitor_unref(mon);
udev_unref(udev);
return 0;
}
```
该代码使用了libudev库来创建udev实例和监视器,并设置要监听的事件类型。在主循环中,它使用select()函数等待事件并处理。当有USB设备插入或拔出时,程序会输出设备的动作和系统名称,并且会创建一个工作线程来处理这些事件。
阅读全文
相关推荐










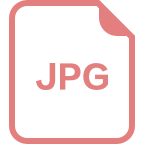
