java用面向对象思想设计编程实现手机生产过程,手机模板产生手机及手机功能实现
时间: 2024-06-08 19:06:18 浏览: 17
好的,下面是一个简单的Java面向对象的手机生产过程设计:
首先,定义一个手机模板类,包含手机的基本信息和方法:
```java
public class PhoneTemplate {
private String brand;
private String model;
private String color;
private String screenSize;
private String batteryCapacity;
private boolean hasCamera;
public PhoneTemplate(String brand, String model, String color, String screenSize, String batteryCapacity, boolean hasCamera) {
this.brand = brand;
this.model = model;
this.color = color;
this.screenSize = screenSize;
this.batteryCapacity = batteryCapacity;
this.hasCamera = hasCamera;
}
public void assemble() {
System.out.println("Assembling " + brand + " " + model + "...");
}
public void test() {
System.out.println("Testing " + brand + " " + model + "...");
}
public void pack() {
System.out.println("Packing " + brand + " " + model + "...");
}
public void printInfo() {
System.out.println("Brand: " + brand);
System.out.println("Model: " + model);
System.out.println("Color: " + color);
System.out.println("Screen Size: " + screenSize);
System.out.println("Battery Capacity: " + batteryCapacity);
System.out.println("Has Camera: " + hasCamera);
}
}
```
接下来,定义一个手机工厂类,用于生产手机:
```java
public class PhoneFactory {
public PhoneTemplate produce(String brand, String model, String color, String screenSize, String batteryCapacity, boolean hasCamera) {
PhoneTemplate phone = new PhoneTemplate(brand, model, color, screenSize, batteryCapacity, hasCamera);
phone.assemble();
phone.test();
phone.pack();
return phone;
}
}
```
最后,定义一个手机类,继承自手机模板类,并添加具体的手机功能:
```java
public class Phone extends PhoneTemplate {
private String operatingSystem;
private int storageCapacity;
private boolean hasWiFi;
public Phone(String brand, String model, String color, String screenSize, String batteryCapacity, boolean hasCamera, String operatingSystem, int storageCapacity, boolean hasWiFi) {
super(brand, model, color, screenSize, batteryCapacity, hasCamera);
this.operatingSystem = operatingSystem;
this.storageCapacity = storageCapacity;
this.hasWiFi = hasWiFi;
}
public void makeCall(String number) {
System.out.println("Making a call to " + number + " using " + brand + " " + model);
}
public void sendText(String number, String message) {
System.out.println("Sending a text to " + number + " using " + brand + " " + model + ": " + message);
}
public void browseWeb(String url) {
if (hasWiFi) {
System.out.println("Browsing the web using " + brand + " " + model + " on " + url);
} else {
System.out.println("Cannot browse the web without WiFi");
}
}
// Other phone functions...
}
```
现在,我们可以使用手机工厂类来生产具体的手机,并使用手机类实现具体的手机功能:
```java
public class Main {
public static void main(String[] args) {
PhoneFactory factory = new PhoneFactory();
Phone iphone = (Phone) factory.produce("Apple", "iPhone X", "Silver", "5.8 inches", "2716 mAh", true, "iOS", 64, true);
iphone.printInfo();
iphone.makeCall("1234567890");
iphone.sendText("1234567890", "Hello, world!");
iphone.browseWeb("https://www.google.com");
Phone samsung = (Phone) factory.produce("Samsung", "Galaxy S10", "Black", "6.1 inches", "3400 mAh", true, "Android", 128, true);
samsung.printInfo();
samsung.makeCall("1234567890");
samsung.sendText("1234567890", "Hello, galaxy!");
samsung.browseWeb("https://www.google.com");
}
}
```
输出结果:
```
Assembling Apple iPhone X...
Testing Apple iPhone X...
Packing Apple iPhone X...
Brand: Apple
Model: iPhone X
Color: Silver
Screen Size: 5.8 inches
Battery Capacity: 2716 mAh
Has Camera: true
Making a call to 1234567890 using Apple iPhone X
Sending a text to 1234567890 using Apple iPhone X: Hello, world!
Browsing the web using Apple iPhone X on https://www.google.com
Assembling Samsung Galaxy S10...
Testing Samsung Galaxy S10...
Packing Samsung Galaxy S10...
Brand: Samsung
Model: Galaxy S10
Color: Black
Screen Size: 6.1 inches
Battery Capacity: 3400 mAh
Has Camera: true
Making a call to 1234567890 using Samsung Galaxy S10
Sending a text to 1234567890 using Samsung Galaxy S10: Hello, galaxy!
Browsing the web using Samsung Galaxy S10 on https://www.google.com
```
相关推荐
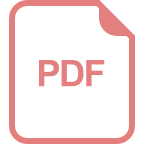
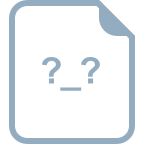
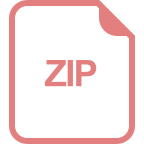














