用mybatis普通开发模式实现以下功能: (1) 创建Product类,对应ssm数据库中product表; (2) 创建映射文件ProductMapper.xml,实现以下功能:查询所有商品信息; (3) 配置mybatis-config.xml (4) 创建测试类
时间: 2024-02-09 12:12:11 浏览: 52
好的,我会根据您的要求来回答问题,以下是答案:
(1)Product类的代码如下:
```
public class Product {
private int id;
private String name;
private double price;
private int stock;
// 省略getter和setter方法
}
```
(2)ProductMapper.xml的代码如下:
```
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.ProductMapper">
<select id="selectAllProducts" resultType="com.example.entity.Product">
SELECT * FROM product
</select>
</mapper>
```
(3)mybatis-config.xml的代码如下:
```
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<typeAlias type="com.example.entity.Product" alias="Product"/>
</typeAliases>
<mappers>
<mapper resource="com/example/mapper/ProductMapper.xml"/>
</mappers>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/ssm"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
</configuration>
```
(4)测试类的代码如下:
```
public class Test {
public static void main(String[] args) {
SqlSession session = null;
try {
// 加载配置文件
InputStream inputStream = Resources.getResourceAsStream("mybatis-config.xml");
SqlSessionFactory sessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
// 创建session
session = sessionFactory.openSession();
// 获取mapper接口的代理对象
ProductMapper productMapper = session.getMapper(ProductMapper.class);
// 查询所有商品信息
List<Product> products = productMapper.selectAllProducts();
for (Product product : products) {
System.out.println(product);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
session.close();
}
}
}
```
以上就是用mybatis普通开发模式实现查询所有商品信息的完整代码。
相关推荐
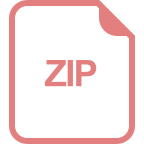
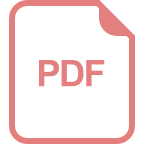
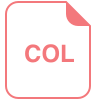
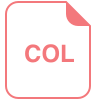
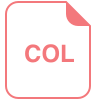
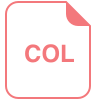
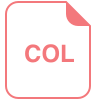









