用ssm做一个添加功能包括把图片的加到数据库里
时间: 2024-03-12 12:49:47 浏览: 124
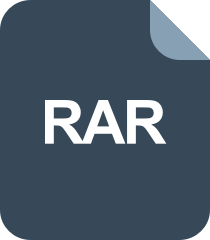
向SQL数据库添加图片
好的,以下是使用 SSM 框架实现添加功能,并将图片保存到数据库中的示例代码:
1. 创建数据库表
创建一个名为 `product` 的表,包含以下字段:
```
id int(11) not null auto_increment primary key,
name varchar(50) not null,
price double not null,
image blob
```
2. 在 `pom.xml` 文件中添加依赖
```xml
<!-- Spring 相关依赖 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<!-- MyBatis 相关依赖 -->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>${mybatis.version}</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>${mybatis.spring.version}</version>
</dependency>
<!-- 数据库驱动依赖 -->
<dependency>
<groupId>com.mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
<!-- Commons FileUpload 依赖 -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.8.0</version>
</dependency>
```
3. 编写实体类 `Product`
```java
public class Product {
private Integer id;
private String name;
private Double price;
private byte[] image;
// getter 和 setter 方法省略
}
```
4. 编写 DAO 接口 `ProductDAO`
```java
public interface ProductDAO {
void insertProduct(Product product);
}
```
5. 编写 DAO 实现类 `ProductDAOImpl`
```java
@Repository
public class ProductDAOImpl implements ProductDAO {
@Autowired
private JdbcTemplate jdbcTemplate;
@Autowired
private SqlSessionFactory sqlSessionFactory;
@Override
public void insertProduct(Product product) {
try (SqlSession sqlSession = sqlSessionFactory.openSession()) {
ProductMapper productMapper = sqlSession.getMapper(ProductMapper.class);
productMapper.insertProduct(product);
sqlSession.commit();
}
}
}
```
6. 编写 MyBatis 映射文件 `ProductMapper.xml`
```xml
<mapper namespace="com.example.dao.ProductMapper">
<insert id="insertProduct" parameterType="com.example.entity.Product">
insert into product (name, price, image) values (#{name}, #{price}, #{image, jdbcType=BLOB})
</insert>
</mapper>
```
7. 编写 Service 接口 `ProductService`
```java
public interface ProductService {
void addProduct(Product product, MultipartFile file) throws IOException;
}
```
8. 编写 Service 实现类 `ProductServiceImpl`
```java
@Service
@Transactional
public class ProductServiceImpl implements ProductService {
@Autowired
private ProductDAO productDAO;
@Override
public void addProduct(Product product, MultipartFile file) throws IOException {
if (!file.isEmpty()) {
product.setImage(file.getBytes());
}
productDAO.insertProduct(product);
}
}
```
9. 编写 Controller 类 `ProductController`
```java
@Controller
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/product/add")
public String showAddForm(Model model) {
model.addAttribute("product", new Product());
return "addProduct";
}
@PostMapping("/product/add")
public String addProduct(@ModelAttribute("product") Product product, @RequestParam("file") MultipartFile file) throws IOException {
productService.addProduct(product, file);
return "redirect:/product/list";
}
}
```
10. 编写表单页面 `addProduct.jsp`
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>添加产品</title>
</head>
<body>
<h1>添加产品</h1>
<form action="${pageContext.request.contextPath}/product/add" method="post" enctype="multipart/form-data">
<label for="name">名称:</label>
<input type="text" name="name" id="name"><br><br>
<label for="price">价格:</label>
<input type="text" name="price" id="price"><br><br>
<label for="file">图片:</label>
<input type="file" name="file" id="file"><br><br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
在这个页面中,表单中包含了产品名称、价格和图片三个字段,使用 `enctype="multipart/form-data"` 属性指定表单提交的数据类型为多部分数据。在提交表单时,控制器会调用 `addProduct()` 方法来将数据保存到数据库中。
以上是一个简单的使用 SSM 框架实现添加功能,并将图片保存到数据库中的示例代码,供参考。
阅读全文
相关推荐
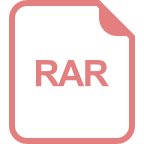
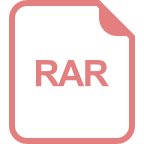
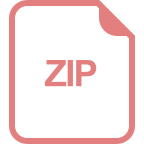
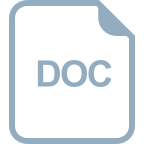


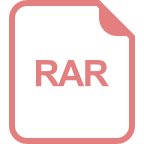
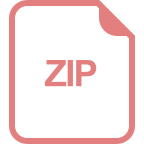
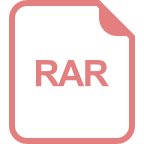
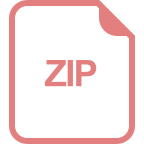
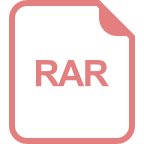
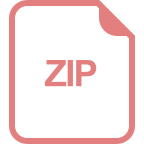
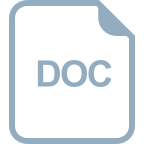
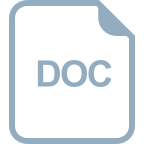
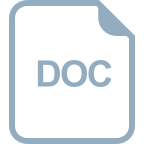
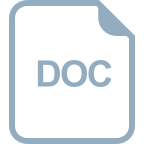
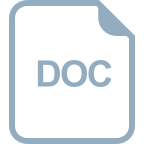