请帮我实现一个完整的目标代码生成器
时间: 2024-03-16 09:41:38 浏览: 90
目标代码生成是编译过程中最后一个阶段,其主要任务是将中间代码转换为目标机器上可执行的机器码。下面是一个简单的目标代码生成器的实现示例:
```python
class CodeGenerator:
def __init__(self, ir):
self.ir = ir
self.code = []
def generate_code(self):
for instr in self.ir:
opcode = instr[0]
if opcode == 'LOAD':
self.load(instr)
elif opcode == 'ADD':
self.add(instr)
elif opcode == 'SUB':
self.sub(instr)
elif opcode == 'MUL':
self.mul(instr)
elif opcode == 'DIV':
self.div(instr)
elif opcode == 'MOV':
self.mov(instr)
elif opcode == 'JMP':
self.jmp(instr)
elif opcode == 'JZ':
self.jz(instr)
elif opcode == 'PRINT':
self.print(instr)
def load(self, instr):
operand1, operand2, result = instr[1], instr[2], instr[3]
self.code.append("MOV %s, [%s]" % (result, operand2))
self.code.append("ADD %s, %s" % (result, operand1))
def add(self, instr):
operand1, operand2, result = instr[1], instr[2], instr[3]
self.code.append("ADD %s, %s" % (result, operand1))
self.code.append("ADD %s, %s" % (result, operand2))
def sub(self, instr):
operand1, operand2, result = instr[1], instr[2], instr[3]
self.code.append("MOV %s, %s" % (result, operand1))
self.code.append("SUB %s, %s" % (result, operand2))
def mul(self, instr):
operand1, operand2, result = instr[1], instr[2], instr[3]
self.code.append("MOV %s, %s" % (result, operand1))
self.code.append("MUL %s, %s" % (result, operand2))
def div(self, instr):
operand1, operand2, result = instr[1], instr[2], instr[3]
self.code.append("MOV %s, %s" % (result, operand1))
self.code.append("DIV %s, %s" % (result, operand2))
def mov(self, instr):
operand, result = instr[1], instr[2]
self.code.append("MOV %s, %s" % (result, operand))
def jmp(self, instr):
label = instr[1]
self.code.append("JMP %s" % label)
def jz(self, instr):
operand, label = instr[1], instr[2]
self.code.append("CMP %s, 0" % operand)
self.code.append("JZ %s" % label)
def print(self, instr):
operand = instr[1]
self.code.append("PUSH %s" % operand)
self.code.append("CALL print")
def get_code(self):
return self.code
```
以上是一个简单的目标代码生成器的实现示例,它支持以下中间代码指令:
- LOAD
- ADD
- SUB
- MUL
- DIV
- MOV
- JMP
- JZ
- PRINT
你可以根据你的具体需求对其进行修改和扩展。
阅读全文
相关推荐
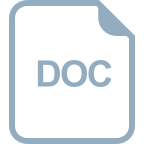
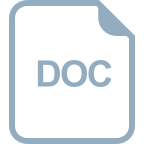
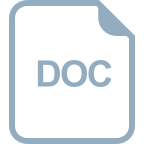
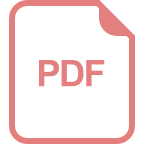
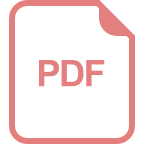
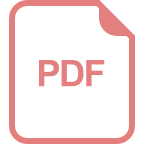
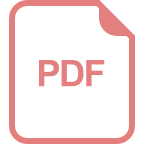
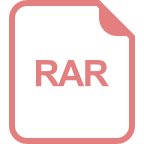
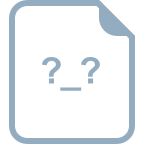
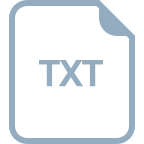
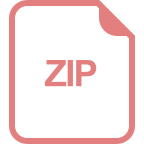
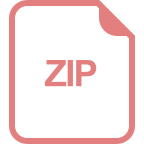
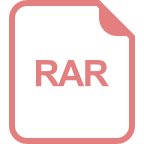
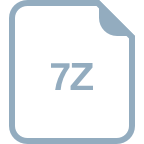
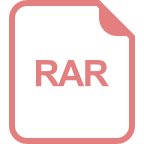
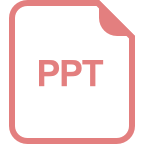
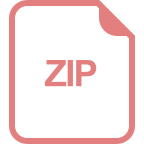
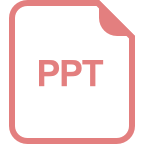
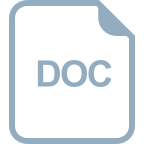