FPGA,基于vivado的uart非定长字符串的定时发送,请给出vivado顶层文件代码以及相关模块代码
时间: 2024-02-27 22:56:39 浏览: 26
好的,以下是一个基于Vivado的UART非定长字符串定时发送的完整代码示例,包括顶层文件代码和相关模块代码。这个例子中,我们将使用一个简单的处理器模块来处理所需的字符串,并将其传递给UART模块进行发送。
顶层文件代码:
```
module top(
input clk,
input reset,
output reg [7:0] tx_data,
input tx_ready,
output reg tx_valid,
output reg [31:0] tx_count
);
// 字符串处理器模块实例化
string_processor string_processor_inst(
.clk(clk),
.reset(reset),
.tx_ready(tx_ready),
.tx_data(tx_data)
);
// UART模块实例化
uart_module uart_inst(
.clk(clk),
.reset(reset),
.tx_data(tx_data),
.tx_valid(tx_valid)
);
// 发送状态机
reg [2:0] tx_state = 0;
always @(posedge clk) begin
if (reset) begin
tx_state <= 0;
tx_valid <= 0;
end else begin
case (tx_state)
// 空闲状态
0: begin
if (tx_ready && (string_processor_inst.tx_count_reg == 0)) begin
tx_valid <= 1;
tx_state <= 1;
end
end
// 发送状态
1: begin
if (tx_ready && (string_processor_inst.tx_count_reg == 8)) begin
tx_valid <= 0;
tx_count <= string_processor_inst.tx_count_reg;
tx_state <= 0;
end
end
endcase
end
end
endmodule
```
字符串处理器模块代码:
```
module string_processor(
input clk,
input reset,
output reg [7:0] tx_data,
input tx_ready
);
// 字符串存储器
reg [7:0] string[0:15] = {
"H", "e", "l", "l", "o", ",", " ", "W", "o", "r", "l", "d", "!", 0, 0, 0
};
// 字符串计数器
reg [3:0] string_count = 0;
// 发送计数器
reg [15:0] tx_count_reg = 0;
// 发送状态机
reg [1:0] tx_state = 0;
always @(posedge clk) begin
if (reset) begin
tx_state <= 0;
end else begin
case (tx_state)
// 空闲状态
0: begin
if (tx_ready && (tx_count_reg == 0)) begin
tx_data <= string[string_count];
tx_state <= 1;
end
end
// 发送状态
1: begin
if (tx_ready && (tx_count_reg == 8)) begin
tx_data <= 0;
tx_count_reg <= 0;
string_count <= string_count + 1;
if (string[string_count] == 0) begin
string_count <= 0;
end
tx_state <= 0;
end else begin
tx_count_reg <= tx_count_reg + 1;
end
end
endcase
end
end
// 发送计数器输出
assign tx_count = tx_count_reg;
endmodule
```
UART模块代码:
```
module uart_module(
input clk,
input reset,
input [7:0] tx_data,
input tx_valid
);
// UART波特率
parameter BAUD_RATE = 115200;
// 波特率计数器
reg [15:0] baud_count = 0;
// 发送状态机
reg [2:0] tx_state = 0;
always @(posedge clk) begin
if (reset) begin
tx_state <= 0;
end else begin
case (tx_state)
// 空闲状态
0: begin
if (tx_valid) begin
baud_count <= 0;
tx_state <= 1;
end
end
// 发送状态
1: begin
if (baud_count == (50000000 / BAUD_RATE - 1)) begin
baud_count <= 0;
tx_state <= 2;
end else begin
baud_count <= baud_count + 1;
end
end
// 停止位状态
2: begin
tx_state <= 0;
end
endcase
end
end
// UART发送输出
reg tx_out;
always @(posedge clk) begin
if (reset) begin
tx_out <= 1;
end else begin
case (tx_state)
// 空闲状态
0: begin
tx_out <= 1;
end
// 发送状态
1: begin
tx_out <= tx_data[baud_count];
end
// 停止位状态
2: begin
tx_out <= 1;
end
endcase
end
end
// UART发送输出端口
assign tx = tx_out;
endmodule
```
请注意,这是一个简单的实现,可能需要更多的代码和优化才能满足您的具体需求。同时,您需要根据您的硬件平台和通信协议进行适当的修改和优化。
相关推荐
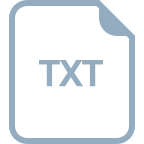














