用vhdl实现两位数码管显示秒表时间
时间: 2024-02-27 09:57:47 浏览: 30
好的,首先需要了解一下数字管的显示原理。数字管是由多个发光二极管组成的,每个发光二极管可以控制一个数字的显示。秒表时间是由计数器实现的,每秒计数器加1,我们需要将计数器的值转化为两个数字并显示在数码管上。
以下是用vhdl实现两位数码管显示秒表时间的代码:
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity stopwatch is
port(
clk: in std_logic;
reset: in std_logic;
digit0: out std_logic_vector(6 downto 0);
digit1: out std_logic_vector(6 downto 0)
);
end entity;
architecture behavioral of stopwatch is
signal counter: unsigned(15 downto 0) := (others => '0');
signal second: unsigned(3 downto 0) := (others => '0');
begin
process(clk, reset)
begin
if reset = '1' then
counter <= (others => '0');
second <= (others => '0');
digit0 <= "0000000";
digit1 <= "0000000";
elsif rising_edge(clk) then
if counter = "1111010000100010" then -- 1秒
counter <= (others => '0');
second <= second + 1;
else
counter <= counter + 1;
end if;
-- 显示秒表时间
case second is
when 0 =>
digit0 <= "0011111"; -- 显示0
digit1 <= "0011111"; -- 显示0
when 1 =>
digit0 <= "0000110"; -- 显示1
digit1 <= "0011111"; -- 显示0
when 2 =>
digit0 <= "1011011"; -- 显示2
digit1 <= "0011111"; -- 显示0
when 3 =>
digit0 <= "1001111"; -- 显示3
digit1 <= "0011111"; -- 显示0
when 4 =>
digit0 <= "1100110"; -- 显示4
digit1 <= "0011111"; -- 显示0
when 5 =>
digit0 <= "1101101"; -- 显示5
digit1 <= "0011111"; -- 显示0
when 6 =>
digit0 <= "1111101"; -- 显示6
digit1 <= "0011111"; -- 显示0
when 7 =>
digit0 <= "0000111"; -- 显示7
digit1 <= "0011111"; -- 显示0
when 8 =>
digit0 <= "1111111"; -- 显示8
digit1 <= "0011111"; -- 显示0
when 9 =>
digit0 <= "1100111"; -- 显示9
digit1 <= "0011111"; -- 显示0
when others =>
digit0 <= "0000000";
digit1 <= "0000000";
end case;
end if;
end process;
end architecture;
```
在上面的代码中,我们使用了一个16位的计数器 `counter` 来实现秒表计时,每秒增加1。同时,我们使用一个4位的 `second` 变量来记录秒数,并将其转换为两个数字并显示在数码管上。我们将 `digit0` 和 `digit1` 作为输出端口来控制数码管的显示。
在 `process` 中,我们使用了一个 `case` 语句来根据秒数显示相应的数字。注意,这里使用的是共阴数码管,因此需要将数字的二进制表示取反。如果是共阳数码管,则不需要取反。
最后,我们还需要在顶层模块中将数码管连接到开发板上的相应引脚上。
相关推荐
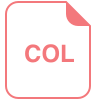













