帮我生产springboot整合netty实现tcp案例
时间: 2023-11-05 16:02:32 浏览: 45
好的,下面是一个简单的Spring Boot整合Netty实现TCP服务器的案例:
1. 创建Spring Boot项目,添加依赖
在pom.xml文件中,添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.65.Final</version>
</dependency>
```
2. 创建Netty服务器
创建一个Netty服务器类,用于接收客户端的TCP连接和消息:
```java
@Component
public class NettyServer {
private final EventLoopGroup bossGroup;
private final EventLoopGroup workerGroup;
private final ServerBootstrap serverBootstrap;
@Autowired
public NettyServer() {
bossGroup = new NioEventLoopGroup();
workerGroup = new NioEventLoopGroup();
serverBootstrap = new ServerBootstrap();
}
@PostConstruct
public void start() throws InterruptedException {
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new NettyServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = serverBootstrap.bind(8080).sync();
f.channel().closeFuture().sync();
}
@PreDestroy
public void stop() {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
```
在上面的代码中,我们使用了@PostConstruct注解,表示在Spring容器初始化之后自动执行start()方法,启动Netty服务器。同时,我们还使用了@PreDestroy注解,表示在Spring容器关闭之前自动执行stop()方法,关闭Netty服务器。
在Netty服务器类中,我们使用了NioEventLoopGroup和ServerBootstrap类来创建一个TCP服务器。我们设置了服务器的监听端口为8080,并在childHandler()方法中添加了一个NettyServerHandler类,用于处理客户端的TCP连接和消息。
3. 创建NettyServerHandler类
创建一个NettyServerHandler类,用于处理客户端的TCP连接和消息:
```java
public class NettyServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
ByteBuf in = (ByteBuf) msg;
try {
String message = in.toString(CharsetUtil.UTF_8);
System.out.println("Received message: " + message);
ctx.write(Unpooled.copiedBuffer("Hello client!", CharsetUtil.UTF_8));
} finally {
ReferenceCountUtil.release(msg);
}
}
@Override
public void channelReadComplete(ChannelHandlerContext ctx) {
ctx.writeAndFlush(Unpooled.EMPTY_BUFFER)
.addListener(ChannelFutureListener.CLOSE);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
```
在NettyServerHandler类中,我们覆盖了ChannelInboundHandlerAdapter类的channelRead()、channelReadComplete()和exceptionCaught()方法。当客户端发送消息时,channelRead()方法会被调用,我们在这个方法中打印出接收到的消息,并向客户端发送一个回复;当客户端的消息接收完成时,channelReadComplete()方法会被调用,我们在这个方法中关闭与客户端的连接;当发生异常时,exceptionCaught()方法会被调用,我们在这个方法中打印出异常信息,并关闭与客户端的连接。
4. 测试
启动Spring Boot应用程序,客户端可以使用telnet命令连接到我们的Netty服务器,例如:
```
telnet localhost 8080
```
输入消息后,服务器将回复"Hello client!"。
以上就是一个简单的Spring Boot整合Netty实现TCP服务器的案例。
相关推荐
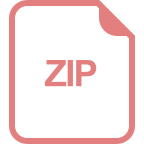
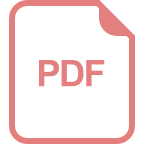














