Kotlin编写一个activity实现背单词页面,显示单词及含义,点击认识按钮切换下一个单词,点击不认识按钮显示单词含义,点击加入生词本,将该单词加入生词本
时间: 2024-01-21 20:02:08 浏览: 168
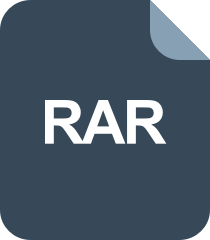
用C++写的一个背单词程序,可供参考

以下是一个使用 Kotlin 编写的 Activity,实现了你所描述的背单词页面功能:
```kotlin
import android.os.Bundle
import android.view.View
import android.widget.Button
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
import java.util.*
class WordActivity : AppCompatActivity() {
private lateinit var wordTextView: TextView
private lateinit var meaningTextView: TextView
private lateinit var knowButton: Button
private lateinit var dontKnowButton: Button
private lateinit var addToVocabularyButton: Button
private val vocabulary = LinkedList<Pair<String, String>>() // 生词本
private val words = listOf( // 单词及其含义
Pair("apple", "苹果"),
Pair("banana", "香蕉"),
Pair("cherry", "樱桃"),
Pair("durian", "榴莲"),
Pair("elderberry", "接骨木"),
Pair("fig", "无花果")
)
private var currentWordIndex = 0 // 当前单词在列表中的下标
private var showMeaning = false // 是否显示单词含义
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_word)
// 初始化控件
wordTextView = findViewById(R.id.word_text_view)
meaningTextView = findViewById(R.id.meaning_text_view)
knowButton = findViewById(R.id.know_button)
dontKnowButton = findViewById(R.id.dont_know_button)
addToVocabularyButton = findViewById(R.id.add_to_vocabulary_button)
// 设置第一个单词
setWord(0)
// 设置按钮点击事件
knowButton.setOnClickListener {
showMeaning = false
setWord(currentWordIndex + 1)
}
dontKnowButton.setOnClickListener {
showMeaning = true
setWord(currentWordIndex)
}
addToVocabularyButton.setOnClickListener {
vocabulary.add(words[currentWordIndex])
}
}
private fun setWord(index: Int) {
currentWordIndex = index
if (currentWordIndex >= words.size) {
// 已经背完了所有单词
wordTextView.text = "Congratulations!"
meaningTextView.visibility = View.GONE
knowButton.isEnabled = false
dontKnowButton.isEnabled = false
addToVocabularyButton.isEnabled = false
} else {
// 显示下一个单词
val (word, meaning) = words[currentWordIndex]
wordTextView.text = word
meaningTextView.text = if (showMeaning) meaning else ""
meaningTextView.visibility = if (showMeaning) View.VISIBLE else View.GONE
addToVocabularyButton.isEnabled = true
}
}
}
```
上面的代码中,`words` 列表存储所有单词及其含义,`vocabulary` 列表存储生词本中的单词。`currentWordIndex` 记录当前单词在列表中的下标,`showMeaning` 记录是否显示单词含义。
在 `onCreate` 方法中,我们通过 `findViewById` 方法获取了所有控件的引用,并设置了第一个单词。同时,我们也设置了按钮的点击事件,分别用于切换下一个单词、显示单词含义、将单词加入生词本。
在 `setWord` 方法中,我们根据 `currentWordIndex` 获取当前单词及其含义,并根据 `showMeaning` 决定是否显示单词含义。如果已经背完了所有单词,我们将显示一个“Congratulations!”的文本,并禁用所有按钮。
在 `addToVocabularyButton` 的点击事件中,我们向 `vocabulary` 列表中添加了当前单词。你可以在需要的地方使用这个列表,比如在一个生词本页面中显示所有已添加的单词。
阅读全文
相关推荐
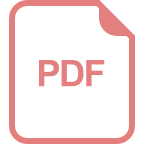
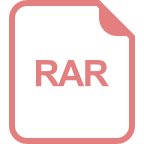
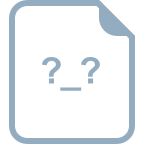
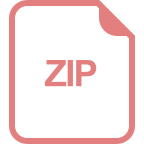
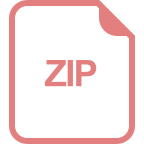
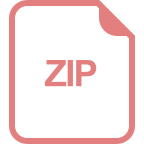
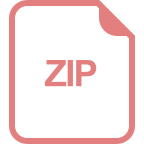
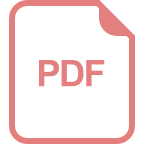
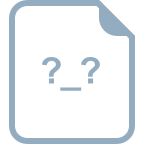
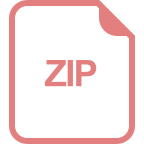
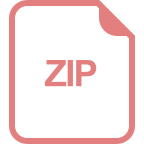
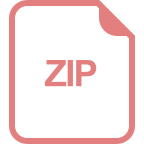
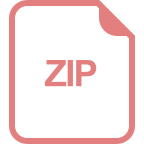
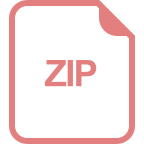
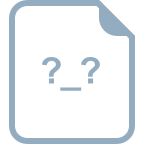
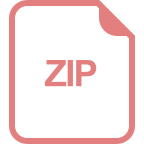
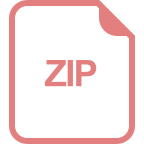