用c++编写程序,将两个字符串连接起来,不使用strcat()函数
时间: 2023-12-15 12:10:36 浏览: 31
你可以使用C++中的字符串类`std::string`来连接两个字符串。以下是一个示例程序,它可以将两个字符串连接起来:
```c++
#include <iostream>
#include <string>
int main() {
std::string str1, str2;
std::cout << "Input the first string: ";
std::getline(std::cin, str1);
std::cout << "Input the second string: ";
std::getline(std::cin, str2);
std::string res = str1 + str2;
std::cout << "Result string: " << res << std::endl;
return 0;
}
```
该程序会提示用户输入两个字符串,然后使用`+`操作符将它们连接到一个结果字符串中。注意,我们直接将两个字符串相加,不需要使用循环或其他操作来完成字符串的连接。最后,我们使用`std::cout`输出结果字符串。
相关问题
c++将两个字符串连接起来,不要用strcat函数。
以下是两种C++将两个字符串连接起来的方法,不使用strcat函数:
1.使用循环遍历字符串并逐个复制到新的字符串中:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str1[20], str2[20], result[40];
cin >> str1 >> str2;
int len1 = strlen(str1), len2 = strlen(str2);
for (int i = 0; i < len1; i++) {
result[i] = str1[i];
}
for (int i = 0; i < len2; i++) {
result[len1 + i] = str2[i];
}
result[len1 + len2] = '\0';
cout << result << endl;
return 0;
}
```
2.使用指针遍历字符串并逐个复制到新的字符串中:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str1[20], str2[20], result[40];
cin >> str1 >> str2;
char *p1 = str1, *p2 = str2, *p3 = result;
while (*p1 != '\0') {
*p3++ = *p1++;
}
while (*p2 != '\0') {
*p3++ = *p2++;
}
*p3 = '\0';
cout << result << endl;
return 0;
}
```
用C++将两个字符串连接起来,不要用strcat函数。
可以使用指针来实现字符串拼接,下面是一个例子:
```c
#include <stdio.h>
void my_strcat(char *dest, const char *src) {
while (*dest) {
dest++;
}
while (*src) {
*dest = *src;
dest++;
src++;
}
*dest = '\0';
}
int main() {
char s1[100] = "Hello, ";
char s2[] = "world!";
my_strcat(s1, s2);
printf("%s\n", s1);
return 0;
}
```
这个程序定义了一个名为 `my_strcat` 的函数,该函数接受两个参数:目标字符串和源字符串,将源字符串拼接到目标字符串的末尾。该函数使用两个指针来遍历两个字符串。第一个指针 `dest` 指向目标字符串的末尾,第二个指针 `src` 指向源字符串的开头。然后,逐个将 `src` 指针指向的字符复制到 `dest` 指针指向的位置,并将两个指针都向前移动,直到 `src` 指针指向源字符串的结束符 `\0`。最后,在目标字符串的末尾添加一个结束符 `\0`。
相关推荐
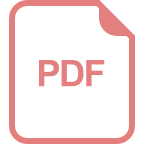
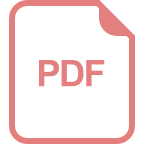
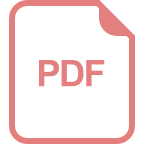












