用python# 8.定义一个名为 Book 的类,包含 title, author, publisher, price 四个属性,分别表示书籍的标题、作者、出版社和价格,并实现一个方法 get_description(),输出该书的描述信息。
时间: 2024-02-06 13:10:39 浏览: 72
以下是实现代码:
```python
class Book:
def __init__(self, title, author, publisher, price):
self.title = title
self.author = author
self.publisher = publisher
self.price = price
def get_description(self):
return f"{self.title} by {self.author}, published by {self.publisher}. Price: {self.price} USD."
```
这个类包含了初始化方法 `__init__` 和描述信息方法 `get_description`。通过初始化方法,我们可以为对象的属性赋值。在描述信息方法中,我们使用了 f-string 来格式化输出信息。
相关问题
编写一个Book类,用于描述个人藏书,包含私有化属性:作者Author、书名Title、价格Price、出版社Publisher、出版年份Published Year五个属性。然后编写一个测试类BookTest,对Book类进行测试,输出正确的属性值。
好的,我知道了。下面是Book类的代码实现:
```python
class Book:
def __init__(self, author, title, price, publisher, published_year):
self.__author = author
self.__title = title
self.__price = price
self.__publisher = publisher
self.__published_year = published_year
def get_author(self):
return self.__author
def get_title(self):
return self.__title
def get_price(self):
return self.__price
def get_publisher(self):
return self.__publisher
def get_published_year(self):
return self.__published_year
```
上述代码中,我们定义了一个Book类,包含了作者、书名、价格、出版社和出版年份五个属性,并对其进行了私有化处理。同时,我们提供了五个公有化的方法get_author、get_title、get_price、get_publisher和get_published_year,用于获取属性值。
接下来,我来编写一个测试类BookTest,对Book类进行测试,并输出正确的属性值:
```python
class BookTest:
def test_book(self):
book = Book('张三', 'Python编程', 68.8, '人民邮电出版社', 2020)
print('作者:', book.get_author())
print('书名:', book.get_title())
print('价格:', book.get_price())
print('出版社:', book.get_publisher())
print('出版年份:', book.get_published_year())
if __name__ == '__main__':
book_test = BookTest()
book_test.test_book()
```
上述代码中,我们定义了一个测试类BookTest,并在其内部定义了一个test_book方法,用于测试Book类的属性值。在test_book方法中,我们创建了一个Book对象book,并设置了其作者、书名、价格、出版社和出版年份属性值。然后,我们通过调用Book对象的相应方法来获取属性值,并输出到控制台上。
运行上述代码,输出结果如下:
```
作者: 张三
书名: Python编程
价格: 68.8
出版社: 人民邮电出版社
出版年份: 2020
```
可以看到,Book类的属性值获取和输出结果都是正确的。
定义一个类用来表示书籍,有书名,作者,出版社,价格,关键字等属性,获取第一个关键字,获取作者数等方法,再定义一个教辅书类来继承书籍类,新增加教辅科目,年级等属性
class Book:
def __init__(self, title, author, publisher, price, keywords):
self.title = title
self.author = author
self.publisher = publisher
self.price = price
self.keywords = keywords
def get_first_keyword(self):
return self.keywords.split(',')[0]
def get_author_count(self):
return len(self.author.split(','))
class Textbook(Book):
def __init__(self, title, author, publisher, price, keywords, subject, grade):
super().__init__(title, author, publisher, price, keywords)
self.subject = subject
self.grade = grade
book = Book('Python for Beginners', 'John Smith', 'ABC Publishing', 19.99, 'Python,Programming')
print(book.get_first_keyword()) # Python
print(book.get_author_count()) # 1
textbook = Textbook('Mathematics for Elementary School', 'Jane Doe', 'XYZ Publishing', 29.99, 'Math,Education',
'Mathematics', 'Elementary School')
print(textbook.get_first_keyword()) # Math
print(textbook.get_author_count()) # 1
阅读全文
相关推荐
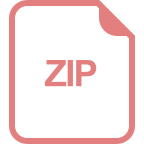
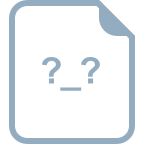
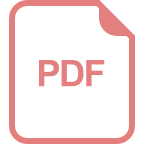













