java发送邮件带附件excel
时间: 2023-07-07 10:04:40 浏览: 90
可以使用JavaMail API来发送带有附件的邮件。下面是一个示例代码:
```java
import java.util.Properties;
import javax.mail.*;
import javax.mail.internet.*;
public class SendEmailWithAttachment {
public static void main(String[] args) {
final String username = "your_email_address";
final String password = "your_email_password";
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("from_address"));
message.setRecipients(Message.RecipientType.TO,
InternetAddress.parse("to_address"));
message.setSubject("Testing Subject");
// 创建一个多部分消息
Multipart multipart = new MimeMultipart();
// 创建文本消息部分
MimeBodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setText("This is message body");
// 添加文本消息部分到多部分消息
multipart.addBodyPart(messageBodyPart);
// 创建附件部分
MimeBodyPart attachPart = new MimeBodyPart();
// 读取附件文件
String filename = "attachment_file_path";
DataSource source = new FileDataSource(filename);
attachPart.setDataHandler(new DataHandler(source));
attachPart.setFileName("attachment_file_name");
// 添加附件部分到多部分消息
multipart.addBodyPart(attachPart);
// 将多部分消息设置为消息内容
message.setContent(multipart);
// 发送消息
Transport.send(message);
System.out.println("邮件已发送");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
```
在代码中,你需要替换以下变量:
- `your_email_address`:你的邮箱地址
- `your_email_password`:你的邮箱密码
- `from_address`:发件人邮箱地址
- `to_address`:收件人邮箱地址
- `attachment_file_path`:附件文件路径
- `attachment_file_name`:附件文件名
注意:在使用JavaMail API发送邮件时,你需要提供你所使用的邮件服务的SMTP服务器地址和端口号。在上面的代码中,我使用的是Gmail的SMTP服务器,如果你使用的是其他邮件服务,请替换相应的SMTP服务器地址和端口号。
阅读全文
相关推荐
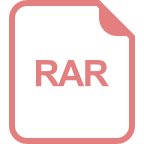
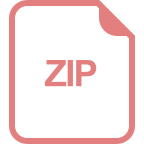
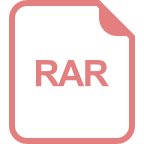
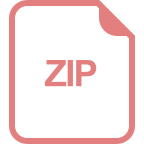


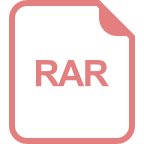
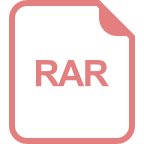
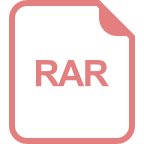
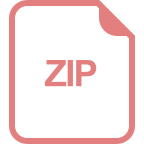
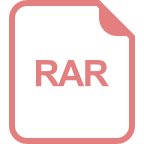
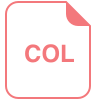


