java 实现带excel发邮件
时间: 2024-05-11 21:13:54 浏览: 85
以下是Java实现带Excel发邮件的示例代码:
```
import java.io.File;
import java.util.Properties;
import javax.activation.DataHandler;
import javax.activation.FileDataSource;
import javax.mail.BodyPart;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
public class SendEmailWithExcel {
public static void main(String[] args) {
String to = "recipient@example.com";
String from = "sender@example.com";
String host = "smtp.example.com";
String subject = "Email with Excel attachment";
// Get system properties
Properties properties = System.getProperties();
// Setup mail server
properties.setProperty("mail.smtp.host", host);
// Get the default Session object.
Session session = Session.getDefaultInstance(properties);
try {
// Create a default MimeMessage object.
MimeMessage message = new MimeMessage(session);
// Set From: header field of the header.
message.setFrom(new InternetAddress(from));
// Set To: header field of the header.
message.addRecipient(Message.RecipientType.TO, new InternetAddress(to));
// Set Subject: header field
message.setSubject(subject);
// Create the message part
BodyPart messageBodyPart = new MimeBodyPart();
// Fill the message
messageBodyPart.setText("This email contains an Excel attachment.");
// Create a multipart message
Multipart multipart = new MimeMultipart();
// Set text message part
multipart.addBodyPart(messageBodyPart);
// Part two is attachment
messageBodyPart = new MimeBodyPart();
String filename = "example.xlsx";
File file = new File(filename);
FileDataSource dataSource = new FileDataSource(file);
messageBodyPart.setDataHandler(new DataHandler(dataSource));
messageBodyPart.setFileName(file.getName());
multipart.addBodyPart(messageBodyPart);
// Send the complete message parts
message.setContent(multipart);
// Send message
Transport.send(message);
System.out.println("Email sent successfully.");
} catch (MessagingException e) {
System.out.println("Failed to send email. Error message: " + e.getMessage());
}
}
}
```
在上面的代码中,我们使用JavaMail API来创建一个带有Excel附件的电子邮件。我们首先设置邮件服务器和收件人、发件人和主题。然后,我们创建一个MimeMessage对象,并使用MimeMultipart对象来创建一个多部分消息。该消息包括一个文本消息和一个Excel附件。最后,我们将消息的内容设置为多部分消息,并使用Transport类的send()方法将其发送。
请注意,为了使此代码正常工作,您需要在classpath中包含JavaMail和Java Activation Framework库。
阅读全文
相关推荐



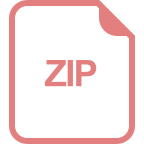

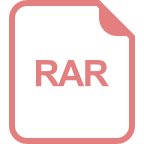






