java字符串工具类示例源代码
时间: 2023-08-18 10:07:43 浏览: 134
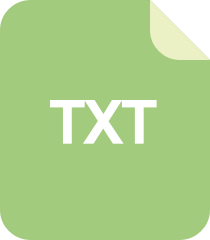
java代码-java字符串工具类源代码合集
### 回答1:
以下是一个 Java 字符串工具类的示例源代码:
```
public class StringUtils {
/**
* 判断字符串是否为空
* @param str 待判断的字符串
* @return true/false
*/
public static boolean isEmpty(String str) {
return str == null || str.length() == ;
}
/**
* 判断字符串是否不为空
* @param str 待判断的字符串
* @return true/false
*/
public static boolean isNotEmpty(String str) {
return !isEmpty(str);
}
/**
* 判断字符串是否为数字
* @param str 待判断的字符串
* @return true/false
*/
public static boolean isNumeric(String str) {
if (isEmpty(str)) {
return false;
}
for (int i = ; i < str.length(); i++) {
if (!Character.isDigit(str.charAt(i))) {
return false;
}
}
return true;
}
/**
* 判断字符串是否为字母
* @param str 待判断的字符串
* @return true/false
*/
public static boolean isLetter(String str) {
if (isEmpty(str)) {
return false;
}
for (int i = ; i < str.length(); i++) {
if (!Character.isLetter(str.charAt(i))) {
return false;
}
}
return true;
}
/**
* 判断字符串是否为汉字
* @param str 待判断的字符串
* @return true/false
*/
public static boolean isChinese(String str) {
if (isEmpty(str)) {
return false;
}
for (int i = ; i < str.length(); i++) {
if (str.charAt(i) < x4e00 || str.charAt(i) > x9fa5) {
return false;
}
}
return true;
}
/**
* 将字符串转换为大写
* @param str 待转换的字符串
* @return 转换后的字符串
*/
public static String toUpperCase(String str) {
if (isEmpty(str)) {
return str;
}
return str.toUpperCase();
}
/**
* 将字符串转换为小写
* @param str 待转换的字符串
* @return 转换后的字符串
*/
public static String toLowerCase(String str) {
if (isEmpty(str)) {
return str;
}
return str.toLowerCase();
}
/**
* 将字符串首字母大写
* @param str 待转换的字符串
* @return 转换后的字符串
*/
public static String capitalize(String str) {
if (isEmpty(str)) {
return str;
}
char firstChar = Character.toUpperCase(str.charAt());
if (str.length() == 1) {
return String.valueOf(firstChar);
}
return firstChar + str.substring(1);
}
/**
* 将字符串首字母小写
* @param str 待转换的字符串
* @return 转换后的字符串
*/
public static String uncapitalize(String str) {
if (isEmpty(str)) {
return str;
}
char firstChar = Character.toLowerCase(str.charAt());
if (str.length() == 1) {
return String.valueOf(firstChar);
}
return firstChar + str.substring(1);
}
}
```
### 回答2:
Java字符串工具类示例源代码如下:
```
public class StringUtil {
/**
* 判断字符串是否为空或null
* @param str 要判断的字符串
* @return 若为空或null,返回true;否则返回false
*/
public static boolean isEmpty(String str) {
return str == null || "".equals(str);
}
/**
* 判断字符串是否为非空
* @param str 要判断的字符串
* @return 若不为空,返回true;否则返回false
*/
public static boolean isNotEmpty(String str) {
return !isEmpty(str);
}
/**
* 去掉字符串两端的空白字符
* @param str 要处理的字符串
* @return 去掉空白字符的新字符串
*/
public static String trim(String str) {
return str == null ? null : str.trim();
}
/**
* 比较两个字符串是否相等,忽略大小写
* @param str1 字符串1
* @param str2 字符串2
* @return 若两个字符串相等,返回true;否则返回false
*/
public static boolean equalsIgnoreCase(String str1, String str2) {
return str1 == null ? str2 == null : str1.equalsIgnoreCase(str2);
}
/**
* 反转字符串
* @param str 要反转的字符串
* @return 反转后的字符串
*/
public static String reverse(String str) {
return str == null ? null : new StringBuilder(str).reverse().toString();
}
}
```
以上是一个简单的Java字符串工具类示例,包含了常见的字符串操作方法,如判断字符串是否为空、去除空白字符、比较字符串是否相等(忽略大小写)、反转字符串等。可以在实际开发中进行改进和扩展,以满足不同的需求。
### 回答3:
下面是一个简单的Java字符串工具类示例源代码:
```java
public class StringUtils {
/**
* 判断字符串是否为空
* @param str 要判断的字符串
* @return true表示字符串为空,false表示字符串不为空
*/
public static boolean isEmpty(String str) {
return str == null || str.length() == 0;
}
/**
* 反转字符串
* @param str 要反转的字符串
* @return 反转后的字符串
*/
public static String reverse(String str) {
if (isEmpty(str)) {
return str;
}
StringBuilder sb = new StringBuilder(str);
return sb.reverse().toString();
}
/**
* 将字符串转换为大写
* @param str 要转换的字符串
* @return 转换后的字符串
*/
public static String toUpperCase(String str) {
if (isEmpty(str)) {
return str;
}
return str.toUpperCase();
}
/**
* 将字符串转换为小写
* @param str 要转换的字符串
* @return 转换后的字符串
*/
public static String toLowerCase(String str) {
if (isEmpty(str)) {
return str;
}
return str.toLowerCase();
}
}
```
以上是一个简单的Java字符串工具类示例,其中包含了判断字符串是否为空、反转字符串、将字符串转换为大写和小写等常用方法。你可以根据自己的需求修改或添加其他方法。
阅读全文
相关推荐
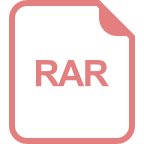
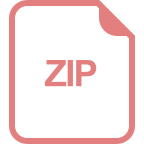
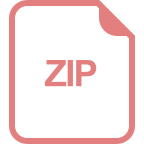
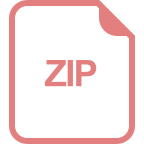
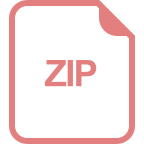
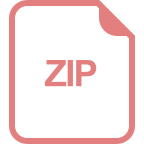
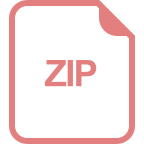
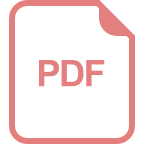
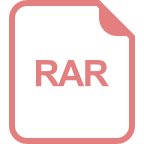
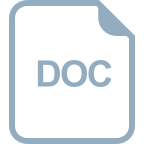
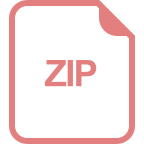
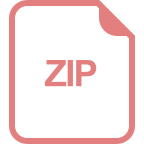
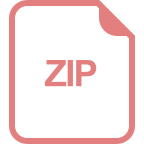
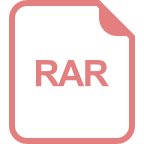
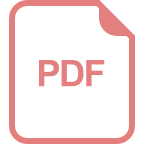
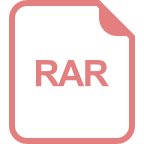