Linux生产者/消费者问题的代码
时间: 2024-08-12 20:07:54 浏览: 44
在Linux中,生产者-消费者问题是一个经典的多线程同步问题,用于演示线程间的通信和互斥访问。通常通过使用互斥锁(mutex)和条件变量(condition variable)来解决。下面是一个简单的Python实现,使用`threading`模块:
```python
import threading
# 假设这是生产者产生的数据队列
queue = []
class Producer(threading.Thread):
def __init__(self, queue, name):
threading.Thread.__init__(self, name=name)
self.queue = queue
def run(self):
while True:
data = "Produced data"
with self.queue.mutex:
if len(self.queue) < 5: # 生产者等待,直到队列不满
self.queue.data.append(data)
self.queue.notify_all() # 唤醒所有消费者
print(f"{self.name} produced {data}")
class Consumer(threading.Thread):
def __init__(self, queue, name):
threading.Thread.__init__(self, name=name)
self.queue = queue
def run(self):
while True:
with self.queue.mutex:
if not self.queue.data: # 消费者等待,直到队列不为空
self.queue.wait() # 等待唤醒
data = self.queue.data.pop(0)
print(f"{self.name} consumed {data}")
# 初始化队列和线程
queue = threading.Condition()
producer = Producer(queue, "Producer")
consumer = Consumer(queue, "Consumer")
# 启动线程
producer.start()
consumer.start()
# 等待线程结束
producer.join()
consumer.join()
```
阅读全文
相关推荐
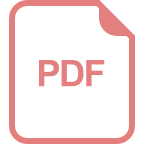
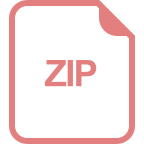
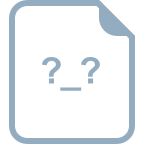
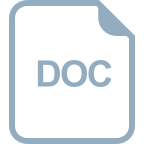
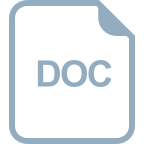
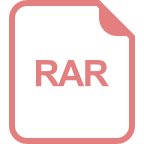
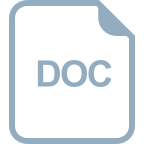
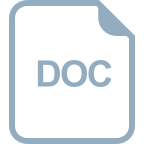
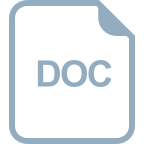
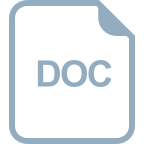
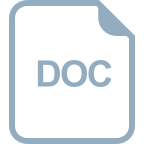
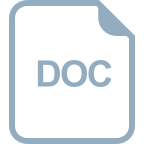
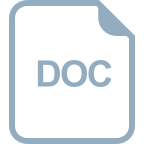
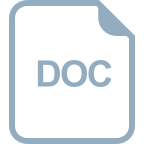
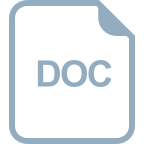
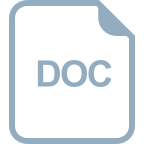
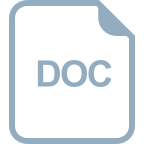
