QWidget右键打开菜单
时间: 2024-06-17 10:02:34 浏览: 104
在Qt中,可以通过以下步骤实现在QWidget上右键打开菜单的功能:
1. 创建一个自定义的QWidget类,例如MyWidget。
2. 在MyWidget的构造函数中设置右键菜单策略为Qt::CustomContextMenu,即:
```
setContextMenuPolicy(Qt::CustomContextMenu);
```
3. 重写MyWidget的contextMenuEvent()函数,该函数会在用户右键点击时触发。在该函数中创建并显示菜单,例如:
```
void MyWidget::contextMenuEvent(QContextMenuEvent *event)
{
QMenu menu(this);
// 添加菜单项
menu.addAction("菜单项1");
menu.addAction("菜单项2");
// 显示菜单
menu.exec(event->globalPos());
}
```
在上述代码中,我们创建了一个QMenu对象,并添加了两个菜单项。然后使用exec()函数在鼠标右键点击的位置显示菜单。
这样,当用户在MyWidget上右键点击时,就会弹出一个包含菜单项的菜单。
相关问题
qtpushbutton右键打开界面
在Qt中,QPushButton(QPushButton)通常用于创建按钮控件,用户点击它会触发预设的动作。如果你想让用户在右键点击QPushButton时打开一个新的界面或弹出菜单,你可以通过连接QPushButton的`rightClicked()`信号到一个槽函数(slot),这个槽函数负责处理右键点击事件并显示你想要的界面。
首先,你需要创建一个槽函数,例如:
```cpp
void on_pushButton_rightClick(QMenu *menu) {
// 创建你想显示的新界面对象
QWidget *newWindow = new QWidget();
// 创建右键菜单
menu->addAction("选项1", []() { newWindow->show(); });
menu->addAction("选项2", []() { /* 进行其他操作 */ });
// 显示菜单
menu->popup(QCursor::pos());
}
```
然后,在你的UI设计中,将`rightClicked`信号链接到这个槽函数:
```cpp
connect(ui->pushButton, &QPushButton::rightClicked, this, &YourClass::on_pushButton_rightClick);
```
在这里,`ui->pushButton`是你的QPushButton对象,`YourClass`是包含上述槽函数的类名。
当用户在QPushButton上右键点击时,会触发`on_pushButton_rightClick`函数,该函数会显示自定义菜单,并允许用户选择相应的操作,如显示新窗口。
qt 重写继承QGraphicsView,打开图像显示时自适应,以原始的图像大小显示鼠标移动的坐标,CTRL和鼠标左键按下可移动图像,鼠标右键出现菜单栏,鼠标滚轮可放大缩小
您可以使用以下代码实现:
```
class CustomGraphicsView : public QGraphicsView
{
public:
CustomGraphicsView(QWidget *parent = nullptr) : QGraphicsView(parent)
{
setDragMode(QGraphicsView::ScrollHandDrag);
setRenderHint(QPainter::Antialiasing);
setRenderHint(QPainter::SmoothPixmapTransform);
setOptimizationFlag(QGraphicsView::DontAdjustForAntialiasing, true);
setViewportUpdateMode(QGraphicsView::FullViewportUpdate);
setTransformationAnchor(QGraphicsView::AnchorUnderMouse);
setResizeAnchor(QGraphicsView::AnchorUnderMouse);
setInteractive(true);
}
protected:
void resizeEvent(QResizeEvent *event) override
{
QGraphicsView::resizeEvent(event);
fitInView(sceneRect(), Qt::KeepAspectRatio);
}
void wheelEvent(QWheelEvent *event) override
{
if (event->modifiers() & Qt::ControlModifier) {
if (event->delta() > 0) {
scale(1.1, 1.1);
} else {
scale(0.9, 0.9);
}
} else {
QGraphicsView::wheelEvent(event);
}
}
void mousePressEvent(QMouseEvent *event) override
{
if (event->button() == Qt::RightButton) {
// show menu
} else {
QGraphicsView::mousePressEvent(event);
}
}
void mouseMoveEvent(QMouseEvent *event) override
{
QPointF scenePos = mapToScene(event->pos());
// display scenePos
QGraphicsView::mouseMoveEvent(event);
}
};
```
这个类继承自 QGraphicsView,实现了自适应、缩放、拖拽、鼠标移动等功能。您可以在您的程序中使用这个类来显示图像。
阅读全文
相关推荐
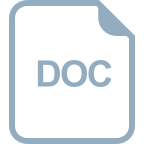
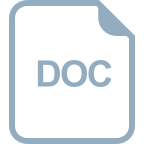
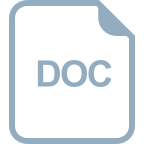
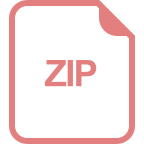
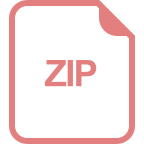
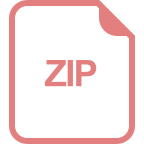










