stm32单片机 让灯从左往右跑 代码
时间: 2024-10-04 14:01:56 浏览: 30
STM32单片机通过GPIO控制LED灯实现从左往右扫描的效果,通常需要使用定时器配合中断来逐位改变LED的状态。这里给出一个简化的示例代码,假设我们有8个LED连接到GPIOA的几个端口,并且使用TIM2定时器进行控制:
```c
#include "stm32f10x.h"
// LED定义
#define LED_PORT GPIOA
#define LED_PIN_1 GPIO_Pin_0
#define LED_PIN_2 GPIO_Pin_1
// ...依此类推直到LED_PIN_8
void led_left_to_right(void) {
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef.TIM_TimeBaseStructure;
// 初始化GPIO
GPIO_InitStructure.GPIO_Pin = LED_PIN_1 | LED_PIN_2 | ... | LED_PIN_8; // 所有LED的组合
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
HAL_GPIO_Init(LED_PORT, &GPIO_InitStructure);
// 初始化定时器TIM2
TIM_TimeBaseInitStructure.TIM_Period = 500; // 设置周期,例如500us
TIM_TimeBaseInitStructure.TIM_ClockDivision = 0;
TIM_TimeBaseInitStructure.TIM_CounterMode = TIM_CounterMode_Up;
HAL_TIM_TimeBaseInit(TIM2, &TIM_TimeBaseInitStructure);
HAL_TIM_EnableCounter(TIM2); // 启动计数
uint8_t direction = 1; // 1表示向右,0表示向左
while (1) {
for (uint8_t i = 0; i < 8; i++) {
if (direction == 1) {
HAL_GPIO_TogglePin(LED_PORT, LED_PIN_7 - i); // 从右到左点亮LED
} else {
HAL_GPIO_TogglePin(LED_PORT, LED_PIN_1 + i * direction); // 从左到右点亮LED
}
// 暂停一段时间以达到扫描效果
HAL_Delay(10); // 10毫秒
}
// 切换方向
direction ^= 1;
}
}
int main(void) {
// ... 其他必要的初始化操作 ...
led_left_to_right();
// 进入死循环
while (1) {}
}
```
这个代码片段是一个基本的框架,实际应用中可能还需要添加中断处理、错误检查等部分。注意这只是一个基础示例,具体硬件配置和引脚定义请按照你的实际情况调整。
阅读全文
相关推荐
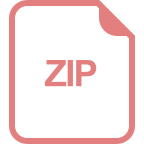
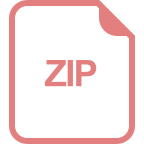


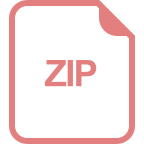
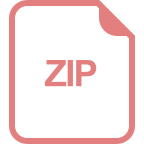






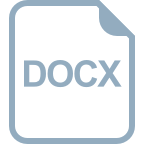
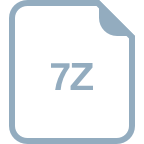