set结构体二分查找
时间: 2023-11-05 08:59:01 浏览: 138
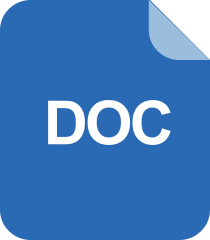
汽车牌照的排序与查找问题
在C++的STL库中,set是一种有序的容器,它使用红黑树实现。由于set是有序的,所以可以使用二分查找算法在set结构体中查找元素。
在C++中,使用STL库的set结构体进行二分查找可以通过调用set的成员函数lower_bound()实现。lower_bound()函数返回一个迭代器,该迭代器指向第一个不小于指定值的元素。如果找不到这样的元素,lower_bound()函数将返回迭代器指向set的end()位置。
以下是使用set结构体进行二分查找的代码示例:
```
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5, 6, 7, 8};
int target1 = 0;
auto it1 = mySet.lower_bound(target1);
if (it1 != mySet.end() && *it1 == target1) {
std::cout << "Element " << target1 << " found at position " << std::distance(mySet.begin(), it1) << std::endl;
} else {
std::cout << "Element " << target1 << " not found in the set." << std::endl;
}
int target2 = 6;
auto it2 = mySet.lower_bound(target2);
if (it2 != mySet.end() && *it2 == target2) {
std::cout << "Element " << target2 << " found at position " << std::distance(mySet.begin(), it2) << std::endl;
} else {
std::cout << "Element " << target2 << " not found in the set." << std::endl;
}
int target3 = 111;
auto it3 = mySet.lower_bound(target3);
if (it3 != mySet.end() && *it3 == target3) {
std::cout << "Element " << target3 << " found at position " << std::distance(mySet.begin(), it3) << std::endl;
} else {
std::cout << "Element " << target3 << " not found in the set." << std::endl;
}
return 0;
}
```
请注意,为了使用set结构体进行二分查找,你需要在程序中包含头文件<set>。
阅读全文
相关推荐
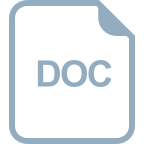
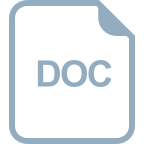
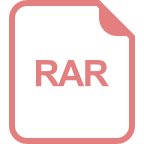
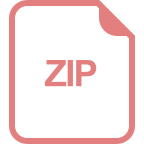
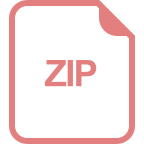
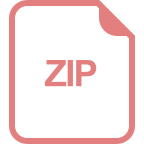
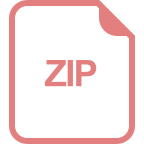
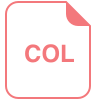
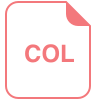
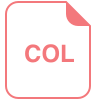
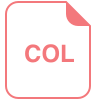
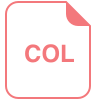
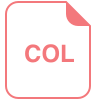




