opencv自动旋转文字
时间: 2023-09-19 11:00:56 浏览: 121
OpenCV可以用来自动旋转文本。在实现自动旋转文本的过程中,可以遵循以下步骤:
1. 导入OpenCV库和必要的辅助库。
``` python
import cv2
import numpy as np
```
2. 读取图像并将其转换为灰度图像。
``` python
image = cv2.imread("image_path.jpg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
3. 对图像进行二值化处理,以便更好地检测文本。
``` python
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
```
4. 检测文本区域并将其拟合成矩形。
``` python
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
rects = [cv2.boundingRect(cnt) for cnt in contours]
```
5. 对于每个检测到的文本矩形,计算其角度并进行旋转。
``` python
for rect in rects:
(x, y, w, h) = rect
center = (x + w // 2, y + h // 2)
# 获取角度
angle = cv2.minAreaRect(contours[0])[-1]
# 对角度进行修正(正负180度)
angle = -angle if angle < -45 else 90 - angle
# 构建旋转矩阵
M = cv2.getRotationMatrix2D(center, angle, 1.0)
# 对图像进行旋转
rotated = cv2.warpAffine(image, M, (image.shape[1], image.shape[0]), flags=cv2.INTER_CUBIC, borderMode=cv2.BORDER_REPLICATE)
# 显示旋转后的图像
cv2.imshow("Rotated Image", rotated)
cv2.waitKey(0)
```
通过以上步骤,我们可以使用OpenCV自动检测并旋转文本。这种方法可以应用于各种场景,例如扫描文档的校正、图像文字识别等。
阅读全文
相关推荐
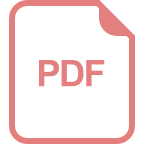
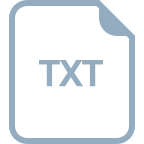
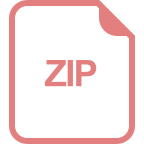
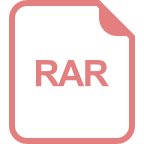
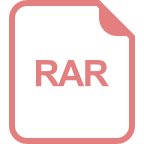
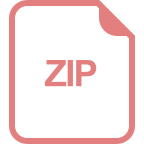
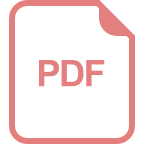
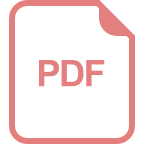
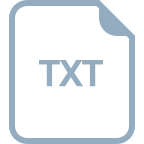
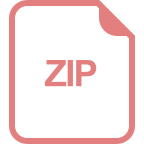
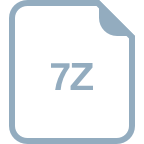
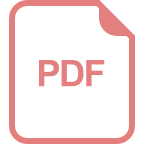
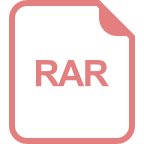
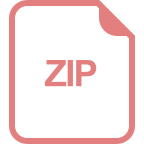
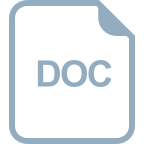
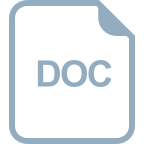