用python写上述
时间: 2024-05-24 17:07:28 浏览: 76
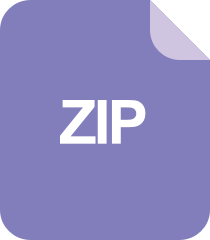
手写数字识别案例_python_手写数字识别_

用Python编写多态的实现方式有以下几种:
1. 虚函数(Virtual Functions):在父类中定义一个虚函数,子类可以根据需要重写该函数。通过父类指针或引用调用虚函数时,会根据实际对象的类型来确定调用哪个子类的函数。
示例代码:
```python
class Animal:
def sound(self):
pass
class Dog(Animal):
def sound(self):
print("汪汪汪")
class Cat(Animal):
def sound(self):
print("喵喵喵")
def make_sound(animal):
animal.sound()
dog = Dog()
cat = Cat()
make_sound(dog) # 输出:汪汪汪
make_sound(cat) # 输出:喵喵喵
```
2. 抽象基类(Abstract Base Classes):使用`abc`模块定义抽象基类,通过继承抽象基类并实现其中的抽象方法来实现多态。
示例代码:
```python
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
print("汪汪汪")
class Cat(Animal):
def sound(self):
print("喵喵喵")
def make_sound(animal):
animal.sound()
dog = Dog()
cat = Cat()
make_sound(dog) # 输出:汪汪汪
make_sound(cat) # 输出:喵喵喵
```
3. 函数重载(Function Overloading):Python中没有直接支持函数重载的特性,但可以通过参数类型判断来实现类似的效果。
示例代码:
```python
class Animal:
def sound(self):
pass
def make_sound(animal):
if isinstance(animal, Dog):
print("汪汪汪")
elif isinstance(animal, Cat):
print("喵喵喵")
class Dog(Animal):
pass
class Cat(Animal):
pass
dog = Dog()
cat = Cat()
make_sound(dog) # 输出:汪汪汪
make_sound(cat) # 输出:喵喵喵
```
阅读全文
相关推荐
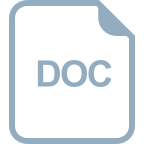
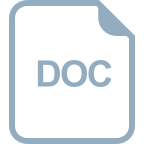
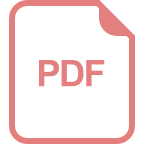
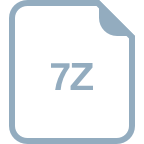
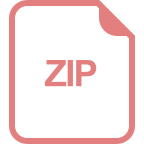
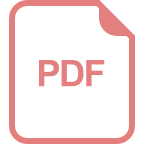


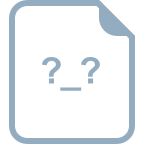
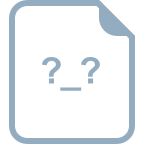
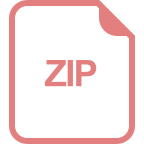
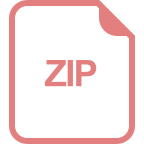
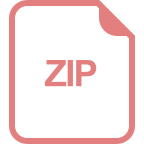
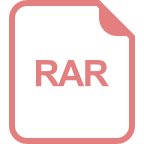
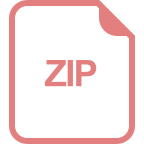
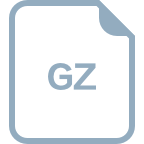
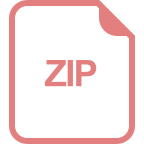
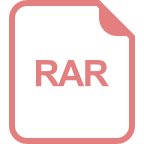