利用所给excel中的温度时间序列,用python绘制时间序列图和直方图,计算数据的平均值和标准差,并用直线标注在前两幅图中
时间: 2024-09-06 16:04:49 浏览: 96
要使用Python对Excel中的温度时间序列数据进行处理和可视化,你可以遵循以下步骤:
1. 读取Excel文件中的数据。
2. 使用matplotlib库绘制时间序列图和直方图。
3. 使用numpy库计算数据的平均值和标准差。
4. 在时间序列图中用直线标注平均值和标准差。
下面是一个简单的代码示例,假设Excel文件名为`temperature_data.xlsx`,温度数据在名为`Sheet1`的工作表中,时间列名为`Time`,温度列名为`Temperature`。
```python
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
# 读取Excel文件中的数据
df = pd.read_excel('temperature_data.xlsx', sheet_name='Sheet1')
# 将时间列转换为pandas的datetime类型(如果需要的话)
df['Time'] = pd.to_datetime(df['Time'])
# 绘制时间序列图
plt.figure(figsize=(10, 5))
plt.plot(df['Time'], df['Temperature'], label='Temperature')
plt.axhline(y=df['Temperature'].mean(), color='r', linestyle='-', label='Average Temperature')
plt.axhline(y=df['Temperature'].std(), color='g', linestyle='-', label='Std. Deviation')
plt.title('Temperature Time Series')
plt.xlabel('Time')
plt.ylabel('Temperature')
plt.legend()
plt.show()
# 绘制直方图
plt.figure(figsize=(10, 5))
plt.hist(df['Temperature'], bins=30, color='blue', alpha=0.7)
plt.axvline(x=df['Temperature'].mean(), color='r', linestyle='-', label='Average Temperature')
plt.axvline(x=df['Temperature'].std(), color='g', linestyle='-', label='Std. Deviation')
plt.title('Temperature Histogram')
plt.xlabel('Temperature')
plt.ylabel('Frequency')
plt.legend()
plt.show()
# 计算平均值和标准差
average_temperature = df['Temperature'].mean()
std_deviation = df['Temperature'].std()
print(f"平均温度: {average_temperature}")
print(f"标准差: {std_deviation}")
```
确保你已经安装了`pandas`、`matplotlib`和`openpyxl`(用于读取Excel文件)库。如果没有安装,可以使用pip进行安装:
```bash
pip install pandas matplotlib openpyxl
```
阅读全文
相关推荐
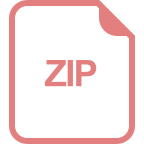
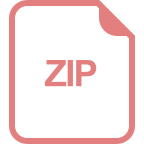
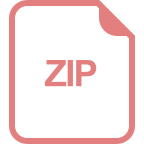















