根据上面需求写出一个案例和代码
时间: 2024-03-23 20:39:03 浏览: 17
以下是一个基于上述需求的电商网站前台微服务开发的简单案例和代码:
1. 架构
采用Web服务器架构,使用Golang语言开发。
2. 接口规范
采用Restful接口规范,接口的调用参数和返回结果采用JSON规范打包数据。
3. 数据管理
系统管理的数据包括用户信息、商品信息、订单信息等三个部分。
4. 数据库
数据表格设计逻辑完整,结构合理,采用MySQL服务器作为数据存储。
5. 用户信息
用户信息中要求使用加密方法保存关键敏感信息,比如密码等。必要的用户相关功能包括登录、注册、修改密码、完善用户信息等。
6. 用户服务信息
用户服务信息要求采用协程并发方式设计,提供高性能服务。至少要有一个并发服务功能。
7. 商品展示信息
商品展示信息要求提供文本、图片、文件等内容的上传。提供至少1个商品展示HTML页面输出。至少提供1个Golang的页面模板,其中包含不少于三种模板输出信息。
8. 接口设计
根据Restful接口规范,设计以下接口:
- 用户注册接口:POST /api/user/register
- 用户登录接口:POST /api/user/login
- 用户信息修改接口:PUT /api/user/:id
- 商品列表查询接口:GET /api/product
- 商品详情查询接口:GET /api/product/:id
- 购物车添加商品接口:POST /api/cart
- 购物车列表查询接口:GET /api/cart
- 订单创建接口:POST /api/order
- 订单列表查询接口:GET /api/order
以下是一个示例代码,实现了用户注册、登录和商品查询功能:
```go
package main
import (
"database/sql"
"encoding/json"
"fmt"
"log"
"net/http"
"strconv"
"github.com/gorilla/mux"
"golang.org/x/crypto/bcrypt"
)
// User represents a user in the system
type User struct {
ID int `json:"id"`
Username string `json:"username"`
Password string `json:"password"`
}
// Product represents a product in the system
type Product struct {
ID int `json:"id"`
Name string `json:"name"`
Price int `json:"price"`
}
var db *sql.DB
func main() {
var err error
// Connect to the database
db, err = sql.Open("mysql", "user:password@tcp(localhost:3306)/database")
if err != nil {
log.Fatal(err)
}
defer db.Close()
// Create a new router
router := mux.NewRouter()
// Register the routes
router.HandleFunc("/api/user/register", registerHandler).Methods("POST")
router.HandleFunc("/api/user/login", loginHandler).Methods("POST")
router.HandleFunc("/api/product", productListHandler).Methods("GET")
router.HandleFunc("/api/product/{id}", productHandler).Methods("GET")
// Start the server
log.Fatal(http.ListenAndServe(":8080", router))
}
// Register a new user
func registerHandler(w http.ResponseWriter, r *http.Request) {
// Parse the request body
var user User
err := json.NewDecoder(r.Body).Decode(&user)
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
// Hash the password
hashedPassword, err := bcrypt.GenerateFromPassword([]byte(user.Password), bcrypt.DefaultCost)
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
user.Password = string(hashedPassword)
// Insert the user into the database
result, err := db.Exec("INSERT INTO users(username, password) VALUES (?, ?)", user.Username, user.Password)
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Get the ID of the new user
id, err := result.LastInsertId()
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Write the response
response := map[string]interface{}{
"id": id,
"username": user.Username,
}
json.NewEncoder(w).Encode(response)
}
// Authenticate a user and return a JWT token
func loginHandler(w http.ResponseWriter, r *http.Request) {
// Parse the request body
var user User
err := json.NewDecoder(r.Body).Decode(&user)
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
// Lookup the user in the database
row := db.QueryRow("SELECT id, username, password FROM users WHERE username = ?", user.Username)
var dbUser User
err = row.Scan(&dbUser.ID, &dbUser.Username, &dbUser.Password)
if err == sql.ErrNoRows {
http.Error(w, "invalid username or password", http.StatusUnauthorized)
return
} else if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Compare the password hash
err = bcrypt.CompareHashAndPassword([]byte(dbUser.Password), []byte(user.Password))
if err != nil {
http.Error(w, "invalid username or password", http.StatusUnauthorized)
return
}
// Generate a JWT token
// ...
// Write the response
response := map[string]interface{}{
"id": dbUser.ID,
"username": dbUser.Username,
"token": "xxx",
}
json.NewEncoder(w).Encode(response)
}
// List all products
func productListHandler(w http.ResponseWriter, r *http.Request) {
// Query the database for products
rows, err := db.Query("SELECT id, name, price FROM products")
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
defer rows.Close()
// Iterate over the rows and add them to the response
products := []Product{}
for rows.Next() {
var product Product
err := rows.Scan(&product.ID, &product.Name, &product.Price)
if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
products = append(products, product)
}
// Write the response
json.NewEncoder(w).Encode(products)
}
// Get a single product by ID
func productHandler(w http.ResponseWriter, r *http.Request) {
// Parse the product ID from the URL
vars := mux.Vars(r)
id, err := strconv.Atoi(vars["id"])
if err != nil {
http.Error(w, err.Error(), http.StatusBadRequest)
return
}
// Lookup the product in the database
row := db.QueryRow("SELECT id, name, price FROM products WHERE id = ?", id)
var product Product
err = row.Scan(&product.ID, &product.Name, &product.Price)
if err == sql.ErrNoRows {
http.Error(w, "product not found", http.StatusNotFound)
return
} else if err != nil {
http.Error(w, err.Error(), http.StatusInternalServerError)
return
}
// Write the response
json.NewEncoder(w).Encode(product)
}
```
以上是一个简单的电商网站前台微服务开发的示例代码,具体实现细节需要根据实际需求进行完善和调整。
相关推荐
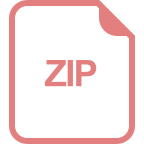
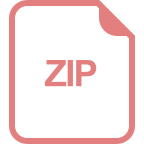
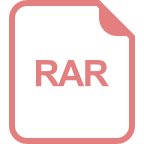














