使用ScheduledExecutorService中的scheduleAtFixedRate使用多任务异步调用并返回结果示例
时间: 2023-07-25 08:27:07 浏览: 122
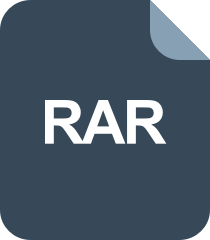
异步调用实例
下面是一个使用`ScheduledExecutorService`中的`scheduleAtFixedRate`方法进行多任务异步调用并返回结果的示例代码:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
public class ScheduleAtFixedRateExample {
public static void main(String[] args) {
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
List<CompletableFuture<Integer>> futures = new ArrayList<>();
// scheduleAtFixedRate方法每隔1秒执行一次任务
executor.scheduleAtFixedRate(() -> {
System.out.println("开始执行任务");
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
// 模拟耗时操作
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return 1;
});
futures.add(future);
}, 0, 1, TimeUnit.SECONDS);
// 每隔0.5秒检查一次所有任务是否完成
ScheduledFuture<?> checkFuture = executor.scheduleAtFixedRate(() -> {
boolean allDone = futures.stream().allMatch(CompletableFuture::isDone);
if (allDone) {
System.out.println("所有任务都已完成");
futures.forEach(future -> {
try {
System.out.println("任务返回结果:" + future.get());
} catch (Exception e) {
e.printStackTrace();
}
});
executor.shutdown();
}
}, 0, 500, TimeUnit.MILLISECONDS);
}
}
```
上述代码中,使用`ScheduledExecutorService`创建一个线程池,并调用`scheduleAtFixedRate`方法定时执行任务。每次任务执行完后,将其返回的`CompletableFuture`对象添加到一个列表中。另外,还有一个定时任务每隔0.5秒检查一次所有任务是否完成,如果都完成了,则打印所有任务的返回结果,并关闭线程池。需要注意的是,在使用`ScheduledExecutorService`时,需要显式地关闭线程池以释放资源。
阅读全文
相关推荐
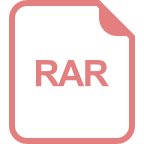
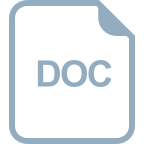
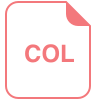
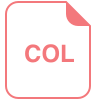
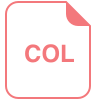
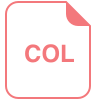
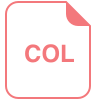
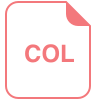
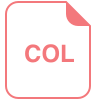
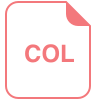
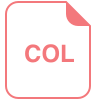
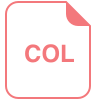
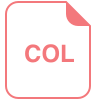
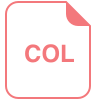
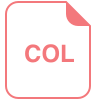
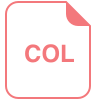