Python1、读入指定彩色图像flower1,分别读取并显示R、G、B各层,用学习过的基于HSI的方法将图像中的花朵分割出来,并将分割结果进行显示。
时间: 2024-03-23 19:40:00 浏览: 54
好的,那么接下来我将为您提供Python代码实现。首先需要安装OpenCV库,您可以通过以下命令进行安装:
```
pip install opencv-python
```
然后,您可以按照以下步骤进行操作:
1. 读入指定彩色图像flower1,并显示原图像:
```python
import cv2
img = cv2.imread('flower1.jpg')
cv2.imshow('Original Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. 分别读取并显示R、G、B各层:
```python
B, G, R = cv2.split(img)
cv2.imshow('Red', R)
cv2.imshow('Green', G)
cv2.imshow('Blue', B)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
3. 将RGB图像转换为HSI图像,并进行阈值分割:
```python
import numpy as np
# 将RGB图像转换为HSI图像
def rgb2hsi(img):
img = img.astype(np.float) / 255.0
B, G, R = cv2.split(img)
I = (R + G + B) / 3.0
S = 1 - np.minimum(np.minimum(R, G), B) / I
H = np.zeros_like(I)
for i in range(img.shape[0]):
for j in range(img.shape[1]):
if R[i, j] == G[i, j] == B[i, j]:
H[i, j] = 0
else:
theta = np.arccos((0.5 * ((R[i, j] - G[i, j]) + (R[i, j] - B[i, j]))) /
np.sqrt((R[i, j] - G[i, j])**2 + (R[i, j] - B[i, j])*(G[i, j] - B[i, j])))
if B[i, j] <= G[i, j]:
H[i, j] = theta
else:
H[i, j] = 2 * np.pi - theta
H = H / (2 * np.pi)
return np.dstack((H, S, I))
# 阈值分割
def threshold(img, h_min, h_max, s_min, s_max, i_min, i_max):
h, s, i = cv2.split(img)
mask = np.zeros_like(h, dtype=np.uint8)
mask[((h >= h_min) & (h <= h_max)) & ((s >= s_min) & (s <= s_max)) & ((i >= i_min) & (i <= i_max))] = 255
return mask
# 分割花朵
img_hsi = rgb2hsi(img)
mask = threshold(img_hsi, 0.7, 1.0, 0.2, 1.0, 0.3, 1.0)
cv2.imshow('Segmented Image', mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`rgb2hsi`函数将RGB图像转换为HSI图像,`threshold`函数进行阈值分割,`mask`即为分割后的结果。
您可以根据实际需要调整阈值范围,以便得到更好的分割效果。
阅读全文
相关推荐
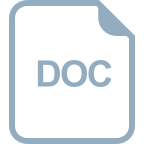
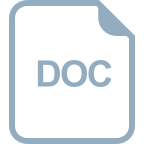
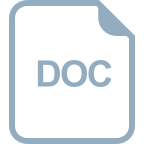

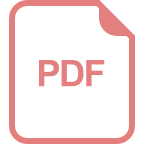













