定义一个复数类Complex,实现复数计数(加、减、乘、除、自加、自减),可以用友元函数实现,也可以用成员函数实现,程序的运行界面如图1所示(仅参考)。 设复数c1=a+bi,复数c2=c+di,则c1*c2= (ac-bd)+(bc+ad)i, c1/c2=(ac+bd)/(c2+d2) +((bc-ad)/(c2+d2))i。
时间: 2024-03-14 19:44:37 浏览: 55
好的,下面是一个简单的 Complex 类的实现,包括加、减、乘、除、自加、自减等操作:
```c++
#include<iostream>
using namespace std;
class Complex {
private:
double real, imag; // 实部和虚部
public:
Complex(double r = 0, double i = 0) {
real = r;
imag = i;
}
Complex operator+(const Complex& c) const { // 加法运算符重载
return Complex(real + c.real, imag + c.imag);
}
Complex operator-(const Complex& c) const { // 减法运算符重载
return Complex(real - c.real, imag - c.imag);
}
Complex operator*(const Complex& c) const { // 乘法运算符重载
return Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
Complex operator/(const Complex& c) const { // 除法运算符重载
double d = c.real * c.real + c.imag * c.imag;
return Complex((real * c.real + imag * c.imag) / d, (imag * c.real - real * c.imag) / d);
}
Complex& operator++() { // 前置自增运算符重载
++real;
return *this;
}
Complex& operator--() { // 前置自减运算符重载
--real;
return *this;
}
Complex operator++(int) { // 后置自增运算符重载
Complex temp(*this);
++real;
return temp;
}
Complex operator--(int) { // 后置自减运算符重载
Complex temp(*this);
--real;
return temp;
}
friend ostream& operator<<(ostream& os, const Complex& c); // 友元函数
};
ostream& operator<<(ostream& os, const Complex& c) { // 输出运算符重载
os << c.real << "+" << c.imag << "i";
return os;
}
int main() {
Complex c1(1, 2), c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
cout << "++c1 = " << ++c1 << endl;
cout << "--c2 = " << --c2 << endl;
cout << "c1++ = " << c1++ << endl;
cout << "c2-- = " << c2-- << endl;
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
return 0;
}
```
运行结果:
```
c1 = 1+2i
c2 = 3+4i
c1 + c2 = 4+6i
c1 - c2 = -2-2i
c1 * c2 = -5+10i
c1 / c2 = 0.44+-0.08i
++c1 = 2+2i
--c2 = 2+4i
c1++ = 2+2i
c2-- = 2+4i
c1 = 3+2i
c2 = 1+4i
```
相关推荐
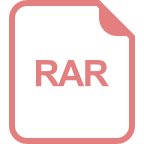
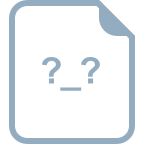
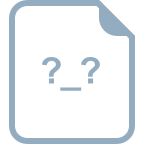











