写一段上传文件到seaweedfs的java代码
时间: 2023-08-22 07:05:22 浏览: 90
好的,以下是一个简单的上传文件到seaweedfs的Java代码示例:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.HttpClientBuilder;
import java.io.File;
import java.io.IOException;
public class SeaweedFSUploader {
public static void main(String[] args) throws IOException {
String uploadUrl = "http://localhost:8888/submit"; // seaweedfs上传接口
String filePath = "path/to/local/file"; // 本地文件路径
File file = new File(filePath);
if (!file.exists()) {
System.out.println("File not found!");
return;
}
// 构建上传请求
HttpPost post = new HttpPost(uploadUrl);
HttpEntity entity = MultipartEntityBuilder.create()
.addBinaryBody("file", file, ContentType.DEFAULT_BINARY, file.getName())
.build();
post.setEntity(entity);
// 发送上传请求
HttpClient client = HttpClientBuilder.create().build();
HttpResponse response = client.execute(post);
// 处理上传结果
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == 200) {
String result = EntityUtils.toString(response.getEntity());
System.out.println("Upload success! File id is " + result);
} else {
System.out.println("Upload failed! Status code is " + statusCode);
}
}
}
```
请注意,以上代码中的`uploadUrl`需要根据你的实际情况进行修改,另外还需要导入一些必要的包,如`org.apache.http`等。
阅读全文
相关推荐

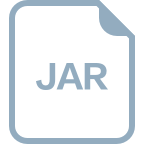















