简单的网络文件共享系统的设计与实现。使用Java代码呈现
时间: 2023-12-12 19:02:15 浏览: 189
网络文件共享系统的设计与实现可以分为以下几个步骤:
1. 设计文件共享协议:定义客户端和服务端之间交互的消息格式和规则。
2. 实现服务端:服务端需要监听客户端的请求,并响应请求。当接收到文件上传请求时,服务端需要将文件保存到指定的位置,并返回上传成功的消息。当接收到文件下载请求时,服务端需要读取指定的文件并将文件数据返回给客户端。
3. 实现客户端:客户端需要向服务端发送请求,并处理服务端响应。当上传文件时,客户端需要将文件数据打包成消息发送给服务端,等待服务端的响应。当下载文件时,客户端需要向服务端发送下载请求,并将接收到的文件数据保存到本地。
以下是一个简单的网络文件共享系统的Java实现:
文件共享协议:
```java
public class Protocol {
// 消息类型常量
public static final int UPLOAD_REQUEST = 1; // 上传请求
public static final int UPLOAD_RESPONSE = 2; // 上传响应
public static final int DOWNLOAD_REQUEST = 3; // 下载请求
public static final int DOWNLOAD_RESPONSE = 4; // 下载响应
// 消息格式:消息类型 + 文件名 + 文件数据
public static byte[] encode(int type, String fileName, byte[] data) throws IOException {
ByteArrayOutputStream out = new ByteArrayOutputStream();
DataOutputStream dataOut = new DataOutputStream(out);
dataOut.writeInt(type);
dataOut.writeUTF(fileName);
dataOut.writeInt(data.length);
dataOut.write(data);
return out.toByteArray();
}
// 解析消息
public static Message decode(byte[] data) throws IOException {
ByteArrayInputStream in = new ByteArrayInputStream(data);
DataInputStream dataIn = new DataInputStream(in);
int type = dataIn.readInt();
String fileName = dataIn.readUTF();
int length = dataIn.readInt();
byte[] fileData = new byte[length];
dataIn.readFully(fileData);
return new Message(type, fileName, fileData);
}
// 消息类
public static class Message {
public int type;
public String fileName;
public byte[] data;
public Message(int type, String fileName, byte[] data) {
this.type = type;
this.fileName = fileName;
this.data = data;
}
}
}
```
服务端:
```java
public class Server {
private ServerSocket serverSocket;
public Server(int port) throws IOException {
serverSocket = new ServerSocket(port);
}
public void start() throws IOException {
while (true) {
Socket socket = serverSocket.accept();
Thread t = new Thread(new ClientHandler(socket));
t.start();
}
}
private class ClientHandler implements Runnable {
private Socket socket;
public ClientHandler(Socket socket) {
this.socket = socket;
}
@Override
public void run() {
try {
InputStream in = socket.getInputStream();
OutputStream out = socket.getOutputStream();
byte[] buffer = new byte[1024];
int len = in.read(buffer);
Protocol.Message request = Protocol.decode(Arrays.copyOf(buffer, len));
if (request.type == Protocol.UPLOAD_REQUEST) { // 处理上传请求
FileOutputStream fileOut = new FileOutputStream(request.fileName);
fileOut.write(request.data);
fileOut.close();
Protocol.Message response = new Protocol.Message(Protocol.UPLOAD_RESPONSE, request.fileName, null);
out.write(Protocol.encode(response.type, response.fileName, response.data));
} else if (request.type == Protocol.DOWNLOAD_REQUEST) { // 处理下载请求
FileInputStream fileIn = new FileInputStream(request.fileName);
byte[] data = new byte[fileIn.available()];
fileIn.read(data);
fileIn.close();
Protocol.Message response = new Protocol.Message(Protocol.DOWNLOAD_RESPONSE, request.fileName, data);
out.write(Protocol.encode(response.type, response.fileName, response.data));
}
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
客户端:
```java
public class Client {
private String serverHost;
private int serverPort;
public Client(String serverHost, int serverPort) {
this.serverHost = serverHost;
this.serverPort = serverPort;
}
public void upload(String fileName) throws IOException {
FileInputStream fileIn = new FileInputStream(fileName);
byte[] data = new byte[fileIn.available()];
fileIn.read(data);
fileIn.close();
Protocol.Message request = new Protocol.Message(Protocol.UPLOAD_REQUEST, fileName, data);
Socket socket = new Socket(serverHost, serverPort);
OutputStream out = socket.getOutputStream();
out.write(Protocol.encode(request.type, request.fileName, request.data));
byte[] buffer = new byte[1024];
int len = socket.getInputStream().read(buffer);
Protocol.Message response = Protocol.decode(Arrays.copyOf(buffer, len));
if (response.type == Protocol.UPLOAD_RESPONSE) {
System.out.println("Upload success: " + response.fileName);
} else {
System.out.println("Upload failed: " + response.fileName);
}
socket.close();
}
public void download(String fileName) throws IOException {
Protocol.Message request = new Protocol.Message(Protocol.DOWNLOAD_REQUEST, fileName, null);
Socket socket = new Socket(serverHost, serverPort);
OutputStream out = socket.getOutputStream();
out.write(Protocol.encode(request.type, request.fileName, request.data));
byte[] buffer = new byte[1024];
int len = socket.getInputStream().read(buffer);
Protocol.Message response = Protocol.decode(Arrays.copyOf(buffer, len));
if (response.type == Protocol.DOWNLOAD_RESPONSE) {
FileOutputStream fileOut = new FileOutputStream(response.fileName);
fileOut.write(response.data);
fileOut.close();
System.out.println("Download success: " + response.fileName);
} else {
System.out.println("Download failed: " + response.fileName);
}
socket.close();
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) throws IOException {
// 启动服务端
Server server = new Server(8000);
server.start();
// 创建客户端并上传、下载文件
Client client = new Client("localhost", 8000);
client.upload("test.txt");
client.download("test.txt");
}
}
```
阅读全文
相关推荐
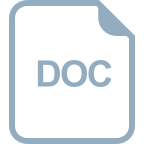
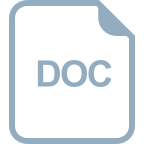
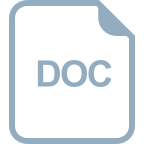
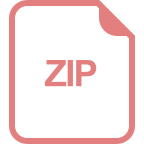
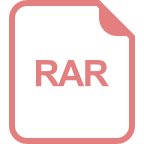
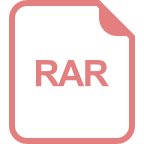
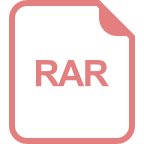
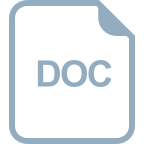
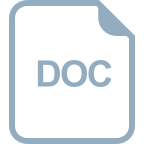
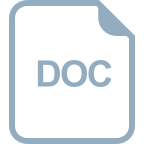
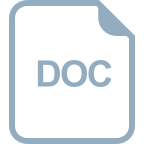
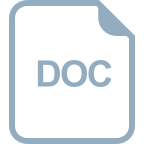
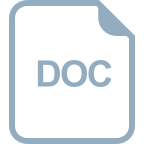
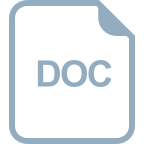
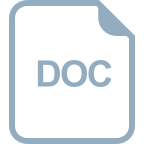
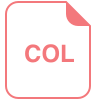
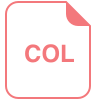
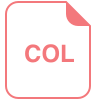
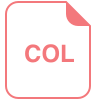