用c语言编写一个词法分析程序、要求能够识别以下代码: int main() { char c=56; int a=1; int bb_b1=7; if(a>bb_b1)c=9; else c=90; while(1)
时间: 2024-01-08 21:04:19 浏览: 21
以下是一个简单的词法分析程序,可以识别部分C语言代码:
```c
#include <stdio.h>
#include <ctype.h>
enum TokenType {
TOKEN_INT, // 整型
TOKEN_CHAR, // 字符
TOKEN_IF, // if
TOKEN_ELSE, // else
TOKEN_WHILE, // while
TOKEN_ASSIGN, // 赋值符号
TOKEN_GT, // 大于号
TOKEN_ID, // 标识符
TOKEN_ERROR, // 错误
TOKEN_EOF // 文件结束
};
struct Token {
enum TokenType type; // token类型
char lexeme[100]; // 词素
};
int main() {
FILE *fp;
char c; // 读入的字符
int i; // 词素下标
struct Token token; // 当前token
fp = fopen("test.txt", "r"); // 打开文件
while ((c = fgetc(fp)) != EOF) { // 读取文件内容
token.type = TOKEN_ERROR; // 初始化token类型为错误
// 判断是否是数字
if (isdigit(c)) {
token.type = TOKEN_INT;
i = 0;
token.lexeme[i++] = c;
while (isdigit(c = fgetc(fp))) {
token.lexeme[i++] = c;
}
token.lexeme[i] = '\0';
ungetc(c, fp); // 将多读的一个字符返回到文件流中
}
// 判断是否是字母
else if (isalpha(c)) {
i = 0;
token.lexeme[i++] = c;
while (isalnum(c = fgetc(fp))) {
token.lexeme[i++] = c;
}
token.lexeme[i] = '\0';
ungetc(c, fp);
// 判断是否是关键字
if (strcmp(token.lexeme, "if") == 0) {
token.type = TOKEN_IF;
} else if (strcmp(token.lexeme, "else") == 0) {
token.type = TOKEN_ELSE;
} else if (strcmp(token.lexeme, "while") == 0) {
token.type = TOKEN_WHILE;
} else {
token.type = TOKEN_ID;
}
}
// 判断是否是赋值符号或大于号
else if (c == '=') {
if ((c = fgetc(fp)) == '=') {
token.type = TOKEN_ERROR;
} else {
token.type = TOKEN_ASSIGN;
ungetc(c, fp);
}
} else if (c == '>') {
token.type = TOKEN_GT;
}
// 判断是否是字符
else if (c == '\'') {
token.type = TOKEN_CHAR;
i = 0;
while ((c = fgetc(fp)) != '\'') {
token.lexeme[i++] = c;
}
token.lexeme[i] = '\0';
}
// 忽略空格、制表符和换行符
else if (isspace(c)) {
continue;
}
// 其他情况为错误
else {
token.type = TOKEN_ERROR;
}
// 输出当前token
printf("%s ", token.lexeme);
switch (token.type) {
case TOKEN_INT:
printf("(int) ");
break;
case TOKEN_CHAR:
printf("(char) ");
break;
case TOKEN_IF:
printf("(if) ");
break;
case TOKEN_ELSE:
printf("(else) ");
break;
case TOKEN_WHILE:
printf("(while) ");
break;
case TOKEN_ASSIGN:
printf("(=) ");
break;
case TOKEN_GT:
printf("(>) ");
break;
case TOKEN_ID:
printf("(id) ");
break;
case TOKEN_ERROR:
printf("(error) ");
break;
}
}
printf("(EOF)\n"); // 输出文件结束标志
fclose(fp); // 关闭文件
return 0;
}
```
该程序会逐个读入文件中的字符,并根据字符类型判断token类型。其中,数字、字母、字符、关键字、赋值符号和大于号均可被识别。空格、制表符和换行符将被忽略。如果出现无法识别的字符,将返回错误类型的token。最后,程序输出每个token的类型和词素,并在文件结束时输出文件结束标志。
相关推荐
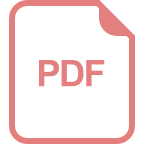
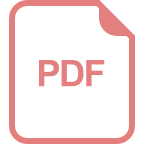
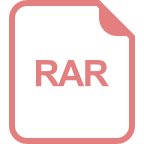














